Operations on matrices
Matlab stands for 'matrix laboratory'. Not surprisingly, matrices, vectors and multidimensional arrays are at the heart of the language. Here we describe how to create, access, modify and otherwise manipulate matrices - the bread and butter of the Matlab programmer.

Creating Matrices
The size of a matrix, transposing a matrix, sums and means, concatenating matrices, basic indexing, logical indexing, linear indexing, reshaping and replication, element-wise matrix arithmetic, matrix multiplication, solving linear systems, more linear algebra, multidimensional arrays, sparse matrices, other numeric data types, other useful functions.
There are a number of ways to create a matrix in Matlab. We begin by simply entering data directly. Entries on each row are separated by a space or comma and rows are separated by semicolons, (or newlines). We say that this matrix is of size 4-by-3 indicating that it has 4 rows and 3 columns. We, (and Matlab) always refer to rows first and columns second.
We can often exploit patterns in the entries to create matrices more succinctly.
We can also create an empty matrix.
Alternatively, there are several functions that will generate matrices for us.
The functions true() and false() , act just like ones() and zeros() but create logical arrays whose entries take only 1 byte each rather than 32.
We can determine the size of a matrix by using the size() command
and the number of elements by using the numel() command.
We refer to dimensions of size 1 as singleton dimensions. The length() command gives the number of elements in the first non-singleton dimension, and is frequently used when the input is a row or column vector; however, it can make code less readable as it fails to make the dimensionality of the input explicit.
We can also determine the size along a specific dimension with size() .
A m-by-n matrix can be transposed into a n-by-m matrix by using the transpose operator '.
You can use the sum() and mean() functions to sum up or take the average of entries along a certain dimension.
The [] argument to the min and max functions indicates that you will specify a dimension.
Matrices can be concatenated by enclosing them inside of square brackets and using either a space or semicolon to specify the dimension. Care must be taken that the matrices are of the right size or Matlab will return an error.
Individual entries can be extracted from a matrix by simply specifying the indices inside round brackets. We can also extract several entries at once by specifying a matrix, or matrices of indices or use the : operator to extract all entries along a certain dimension. The 'end' statement stands for the last index of a dimension.
We can also extract entries using a bit pattern, i.e. a matrix of logical values. Only the entries corresponding to true are returned. This can be particularly useful for selecting elements that satisfy some logical criteria such as being larger than a certain value. We can create a logical matrix by relating a numeric matrix to either a scalar value or matrix of the same size via one of the logical operators, < > <= >= == ~= or by a binary function such as isprime() or isfinite() .
We can then use this logical matrix to extract elements from A. In the following line, we repeat the call to A > 30 but pass the result directly in, without first storing the interim result.
We could also achieve the same result using the find() function, which returns the indices of all of the non-zero elements in a matrix. While this command is useful when the indices themselves are of interest, using find() can be slightly slower than logical indexing although it is a very common code idiom.
We can check that two matrices are equal, (i.e. the same size with the same elements) with the isequal() function. Using the == relation returns a matrix of logical values, not a single value.
Assignment operations, in which we change a value or values in a matrix, are performed in a very similar way to the indexing operations above. Both parallel and logical indexing can be used. We indicate which entries will be changed by performing an indexing operation on the left hand side and then specify the new values on the right hand side. The right must be either a scalar value, or a matrix with the same dimensions as the resulting indexed matrix on the left. Matlab automatically expands scalar values on the right to the correct size.
We can assign every value at once by using the colon operator. The following command temporarily converts A to a column vector, assigns the values on the right hand side and converts back to the original dimensions.
Recall from the indexing section that indices can be repeated returning the corresponding entry multiple times as in A([1,1,1],3). You can also repeat indices in assignments but the results are not what you might expect.
You may have expected the entry A(1,1) to now have a value of 4 instead of 2 since we indexed entry A(1,1) three times. Matlab calculates the right hand side completely before assigning the values and so the value of 2 is simply assigned to A(1,1) three times.
Assigning [] deletes the corresponding entries from the matrix. Only deletions that result in a rectangular matrix are allowed.
When the indices in an assignment operation exceed the size of the matrix, Matlab, rather than giving an error, quietly expands the matrix for you. If necessary, it pads the matrix with zeros. Using this feature is somewhat inefficient, however, as Matlab must reallocate a sufficiently large chunk of contiguous memory and copy the array. It is much faster to preallocate the maximum desired size with the zeros command first, whenever the maximum size is known in advance: see here for details.
When only one dimension is specified in an indexing or assignment operation, Matlab performs linear indexing by counting from top to bottom and then left to right so that the last entry in the first column comes just before the first entry in the second column.
The functions ind2sub() and sub2ind() will convert from a linear index to regular indices and vice versa, respectively. In both cases, you must specify the size of the underlying matrix.
Sometimes, when dealing with multi-dimensional arrays, it is annoying that these functions return/ require multiple separate arguments. We therefore provide the following alternative functions: ind2subv and subv2ind
It is sometimes useful to reshape an array of size m-by-n to size p-by-q where m*n = p*q. The reshape() function lets you do just that. The elements are placed in such a way so as to preserve the order induced by linear indexing. In other words, if a(3) = 3 before reshaping, a(3) will still equal 3 after reshaping.
Further, the repmat() function can be used to tile an array m-by-n times.
We can perform the arithmetical operations, addition, subtraction, multiplication, division, and exponentiation on every element of a matrix. In fact, we can also use functions such as sin() , cos() , tan() , log() , exp() , etc to operate on every entry but we will focus on the former list for now.
If one operand is a scalar value, an element-wise operation is automatically performed. However, if both operands are matrices, a dot must precede the operator as in .* , .^ , ./, and further, both matrices must be the same size.
Matlab also has the .\ operator which is the same as the ./ operator with the order of the operands reversed so that (A ./ B) = (B .\ A). As this is infrequently used, it should be avoided for the sake of clarity.
We can also perform matrix multiplication of an m-by-n matrix and an n-by-p matrix yielding an m-by-p matrix. Suppose we multiple A*B = C, then C(i,j) = A(i,:)*B(:,j) , that is, the dot product of the ith row from A and the jth column from B. The dot, (or inner) product of a and b is just sum(a.*b) . Further, if A is a square matrix, we can multiply A by itself k times by the matrix exponentiation A^k.
Suppose we have the matrix equation Y = XW where X is an n-by-d matrix, W is a d-by-k matrix and thus Y is an n-by-k matrix. If X is invertible, we could solve for W= inv(X)*Y. The inv() function returns the inverse of a matrix. If n ~= d, however, we can still solve for the least squares estimate of W by taking the pseudo inverse of X, namely inv(X'*X)*X', or more concisely using the Matlab function, pinv(X) . Matlab allows you to solve more directly, (and efficiently) for W, (i.e. the lsq estimate of W), however, by using the matrix division X \ Y. Both X and Y must have the same number of rows. (Below we specify a seed to the random number generators so that they return the same values every time this demo is run).
Matlab also supports matrix right division such that X \ Y = (Y' / X')' but as this is infrequently used, it should be avoided for the sake of clarity.
Matlab was original designed primarily as a linear algebra package, and this remains its forte. Here we only list a few functions for brevity, do not display the results. Many functions can also take additional arguments: type doc svd for instance to see documentation for the svd() function.
Numeric matrices in Matlab can extend to an arbitrary number of dimensions, not just 2. We can use the zeros() , ones() , rand() , randn() functions to create n-dimensional matrices by simply specifying n parameters. We can also use repmat() to replicate matrices along any number of dimensions, or the cat() function, which is a generalization of the [] concatenation we saw earlier. Indexing, assignment,and extension work just as before, only with n indices, as opposed to just two. Finally, we can use functions like sum() , mean() , max() or min() by specifying the dimension over which we want the function to operate. sum(A,3) for example, sums over, or marginalizes out, the 3rd dimension.
Taking the mean of say a 4-by-4-by-2-by-2 matrix along the 3rd dimension results in a matrix of size 4-by-4-by-1-by-2. If we want to remove the 3rd singleton dimension, (which is only acting now as a place holder) we can use the squeeze() function.
The ndims() functions indicates how many dimensions an array has. Final singleton dimensions are ignored but singleton dimensions occurring before non-singleton dimensions are not.
The meshgrid() function we saw earlier extends to 3 dimensions. If you need to grid n-dimensional space, use the ndgrid() function but keep in mind that the number of elements grows exponentially with the dimension.
When dealing with large matrices containing many zeros, you can save a great deal of space by using Matlab's sparse matrix construct. Sparse matrices can be used just like ordinary matrices but can be slower depending on the operation. The functions full() and sparse() convert back and forth. Currently Matlab supports double and logical sparse matrices.
The spy() function can be used to visualize the sparsity pattern of a matrix.
The spalloc() function can be used to preallocate space for a sparse matrix. The following command creates a 100-by-100 matrix with room currently for 10 non-zero elements. More than 10 non-zero elements can be added later but this can be slow as Matlab will need to find a larger chunk of memory and copy the non-zero elements.
Matlab has limited support for 11 numeric data types similar to those in the C programming language. Below we create matrices of each type and show the space each matrix requires. Matrices can also be created by using the commands int8() , single() , int64() etc. The cast() command, converts from one data type to another. You can determine the class of a variable with the class() command and the maximum or minimum values each class is able to represent with the intmax() , intmin() , realmax() , and realmin() functions. The uint classes are unsigned and not able to represent negative numbers. Unfortunately many Matlab functions do not support types other than double or logical. Functions such as sum() have an optional parameter 'native', which performs summation without automatically casting to double. To perform variable precision arithmetic, check out the vpa() function available in the symbolic math toolbox.
The cumsum() and cumprod() functions can be useful for generating a running sum or product of an array. The diff() function returns the differences between consecutive elements. You can specify the dimension over which you want them to operate. If you leave this blank, they operate over the first non-singleton dimension.
The histc function is useful for, (among other things) counting the number of occurrences of numbers in an array.
The filter() function can be used to calculate values that depend on previous values in an array. While it is quite a complicated function, here is an easy way to calculate the points halfway between each consecutive point in an array. The first result is just half the value of the first element. You can calculate a running average in which only a window of k elements are included with filter(ones(1,k)/k,1,data).
Published with MATLAB® 7.10
Assigning to Variables and Matrices
Be sure to read the indexing page first.
Assigning a matrix to a variable can be done using the = operator.
To change one entry in a matrix, assign to the indexed value:
If a matrix is not assigned, a sufficiently large matrix is generated.
If a matrix is assigned, but does not contain the element, the matrix is made sufficiently large.
You can assign to a block within a matrix. If the right hand side is a scalar, all values within that block are assigned that value. If the right hand side is a matrix, it must have the same dimensions of the block you are assigning to. The matrix is automatically enlarged if you index outside the matrix.
The colon and end can also be used to denote a row:
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Matlab - Matrix
- MATLAB - Variables
- MATLAB Syntax
- MATLAB syms
- Sparse Matrices in MATLAB
- Find inverse of matrix in MATLAB
- Simulink in MATLAB
- Normalize Data in MATLAB
- Polynomials in MATLAB
- MATLAB - Data Types
- MATLAB - Algebra
- Matrix Formula
- Matrix Addition
- Matrix Algebra in R
- Matrix Data Structure
- Add Matrix in C
- matrices in LaTeX
- Compute before Matrix
- Fill an empty matrix in R
Matlab – Matrix
A Matrix is a two-dimensional array of elements. In MATLAB, the matrix is created by assigning the array elements that are delimited by spaces or commas and using semicolons to mark the end of each row. Now let’s have a glance at some examples to understand it better.
a = [elements; elements]
Example: Creating a Matrix
Output:
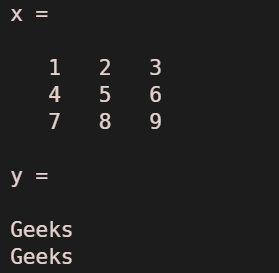
Example: Knowing the size of the Matrix
Output:
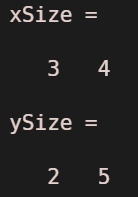
Accessing the elements of a matrix
To reference an element in a matrix, we write matrix(m, n). Here m and n are row and column indexes.
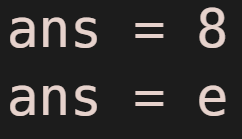
To access multiple elements in the matrix, we write
matrix(x:y;,xy)
Example 2:
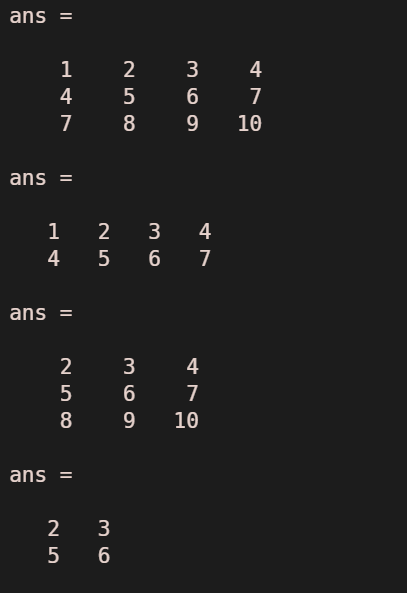
Adding elements/dimensions to a matrix
To add elements/dimension to a matrix we can use one of the following methods:
cat(dimension,A,B,….) or x = [A;B]
In the above syntax, A & B are matrices that we need to pass into the function cat() or concatenate by using square brackets. Here dimension parameter must be either 1 or 2 for table or timetable input.
Example 1:
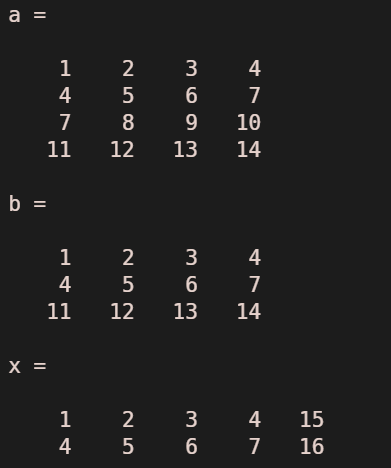
Example 3:
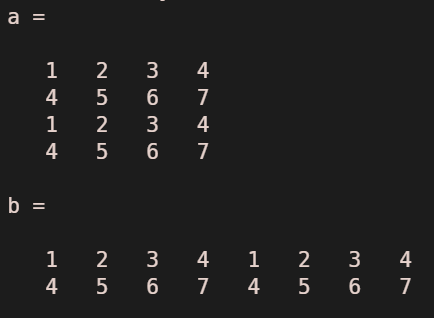
Example 4: Deleting a row/column of a matrix
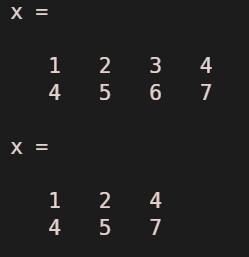
Note:
- Unlike most of the programming languages, MATLAB array indexes start from 1.
- To perform operations(almost all) on the matrices, dimensions must be the same.
- A semicolon must be added at each end of the statement in order to avoid multiple outputs.
- In MATLAB, a string is a character array.
- In MATLAB, length() gives the length of an array, and size() gives the size of a matrix.
Please Login to comment...
Similar reads.
- Computer Subject
- Programming Language
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Help Center Help Center
- Help Center
- Trial Software
- Product Updates
- Documentation
Multidimensional Arrays
A multidimensional array in MATLAB® is an array with more than two dimensions. In a matrix, the two dimensions are represented by rows and columns.
Each element is defined by two subscripts, the row index and the column index. Multidimensional arrays are an extension of 2-D matrices and use additional subscripts for indexing. A 3-D array, for example, uses three subscripts. The first two are just like a matrix, but the third dimension represents pages or sheets of elements.
Creating Multidimensional Arrays
You can create a multidimensional array by creating a 2-D matrix first, and then extending it. For example, first define a 3-by-3 matrix as the first page in a 3-D array.
Now add a second page. To do this, assign another 3-by-3 matrix to the index value 2 in the third dimension. The syntax A(:,:,2) uses a colon in the first and second dimensions to include all rows and all columns from the right-hand side of the assignment.
The cat function can be a useful tool for building multidimensional arrays. For example, create a new 3-D array B by concatenating A with a third page. The first argument indicates which dimension to concatenate along.
Another way to quickly expand a multidimensional array is by assigning a single element to an entire page. For example, add a fourth page to B that contains all zeros.
Accessing Elements
To access elements in a multidimensional array, use integer subscripts just as you would for vectors and matrices. For example, find the 1,2,2 element of A , which is in the first row, second column, and second page of A .
Use the index vector [1 3] in the second dimension to access only the first and last columns of each page of A .
To find the second and third rows of each page, use the colon operator to create your index vector.
Manipulating Arrays
Elements of multidimensional arrays can be moved around in many ways, similar to vectors and matrices. reshape , permute , and squeeze are useful functions for rearranging elements. Consider a 3-D array with two pages.
Reshaping a multidimensional array can be useful for performing certain operations or visualizing the data. Use the reshape function to rearrange the elements of the 3-D array into a 6-by-5 matrix.
reshape operates columnwise, creating the new matrix by taking consecutive elements down each column of A , starting with the first page then moving to the second page.
Permutations are used to rearrange the order of the dimensions of an array. Consider a 3-D array M .
Use the permute function to interchange row and column subscripts on each page by specifying the order of dimensions in the second argument. The original rows of M are now columns, and the columns are now rows.
Similarly, interchange row and page subscripts of M .
When working with multidimensional arrays, you might encounter one that has an unnecessary dimension of length 1. The squeeze function performs another type of manipulation that eliminates dimensions of length 1. For example, use the repmat function to create a 2-by-3-by-1-by-4 array whose elements are each 5, and whose third dimension has length 1.
Use the squeeze function to remove the third dimension, resulting in a 3-D array.
Related Topics
- Creating, Concatenating, and Expanding Matrices
- Array Indexing
- Reshaping and Rearranging Arrays

MATLAB Command
You clicked a link that corresponds to this MATLAB command:
Run the command by entering it in the MATLAB Command Window. Web browsers do not support MATLAB commands.
Select a Web Site
Choose a web site to get translated content where available and see local events and offers. Based on your location, we recommend that you select: .
- Switzerland (English)
- Switzerland (Deutsch)
- Switzerland (Français)
- 中国 (English)
You can also select a web site from the following list:
How to Get Best Site Performance
Select the China site (in Chinese or English) for best site performance. Other MathWorks country sites are not optimized for visits from your location.
- América Latina (Español)
- Canada (English)
- United States (English)
- Belgium (English)
- Denmark (English)
- Deutschland (Deutsch)
- España (Español)
- Finland (English)
- France (Français)
- Ireland (English)
- Italia (Italiano)
- Luxembourg (English)
- Netherlands (English)
- Norway (English)
- Österreich (Deutsch)
- Portugal (English)
- Sweden (English)
- United Kingdom (English)
Asia Pacific
- Australia (English)
- India (English)
- New Zealand (English)
Contact your local office
Help Center Help Center
- Hilfe-Center
- Produkt-Updates
- Documentation
Basic Matrix Operations
This example shows basic techniques and functions for working with matrices in the MATLAB® language.
First, let's create a simple vector with 9 elements called a .
Now let's add 2 to each element of our vector, a , and store the result in a new vector.
Notice how MATLAB requires no special handling of vector or matrix math.
Creating graphs in MATLAB is as easy as one command. Let's plot the result of our vector addition with grid lines.
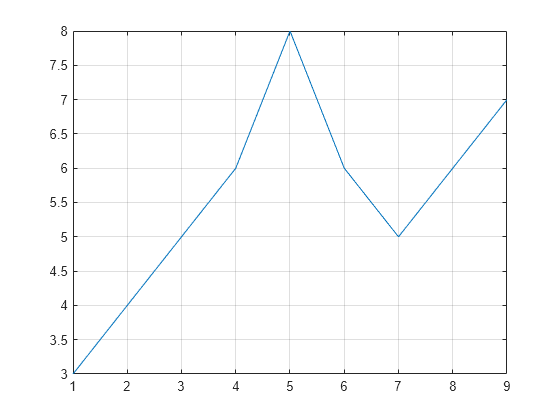
MATLAB can make other graph types as well, with axis labels.

MATLAB can use symbols in plots as well. Here is an example using stars to mark the points. MATLAB offers a variety of other symbols and line types.

One area in which MATLAB excels is matrix computation.
Creating a matrix is as easy as making a vector, using semicolons (;) to separate the rows of a matrix.
We can easily find the transpose of the matrix A .
Now let's multiply these two matrices together.
Note again that MATLAB doesn't require you to deal with matrices as a collection of numbers. MATLAB knows when you are dealing with matrices and adjusts your calculations accordingly.
Instead of doing a matrix multiply, we can multiply the corresponding elements of two matrices or vectors using the .* operator.
Let's use the matrix A to solve the equation, A*x = b. We do this by using the \ (backslash) operator.
Now we can show that A*x is equal to b.
MATLAB has functions for nearly every type of common matrix calculation.
There are functions to obtain eigenvalues ...
... as well as the singular values.
The "poly" function generates a vector containing the coefficients of the characteristic polynomial.
The characteristic polynomial of a matrix A is
d e t ( λ I - A )
We can easily find the roots of a polynomial using the roots function.
These are actually the eigenvalues of the original matrix.
MATLAB has many applications beyond just matrix computation.
To convolve two vectors ...
... or convolve again and plot the result.

At any time, we can get a listing of the variables we have stored in memory using the who or whos command.
You can get the value of a particular variable by typing its name.
You can have more than one statement on a single line by separating each statement with commas or semicolons.
If you don't assign a variable to store the result of an operation, the result is stored in a temporary variable called ans .
As you can see, MATLAB easily deals with complex numbers in its calculations.
Related Topics
- Array vs. Matrix Operations
MATLAB-Befehl
Sie haben auf einen Link geklickt, der diesem MATLAB-Befehl entspricht:
Führen Sie den Befehl durch Eingabe in das MATLAB-Befehlsfenster aus. Webbrowser unterstützen keine MATLAB-Befehle.
Select a Web Site
Choose a web site to get translated content where available and see local events and offers. Based on your location, we recommend that you select: .
- Switzerland (English)
- Switzerland (Deutsch)
- Switzerland (Français)
- 中国 (English)
You can also select a web site from the following list:
How to Get Best Site Performance
Select the China site (in Chinese or English) for best site performance. Other MathWorks country sites are not optimized for visits from your location.
- América Latina (Español)
- Canada (English)
- United States (English)
- Belgium (English)
- Denmark (English)
- Deutschland (Deutsch)
- España (Español)
- Finland (English)
- France (Français)
- Ireland (English)
- Italia (Italiano)
- Luxembourg (English)
- Netherlands (English)
- Norway (English)
- Österreich (Deutsch)
- Portugal (English)
- Sweden (English)
- United Kingdom (English)
Asia Pacific
- Australia (English)
- India (English)
- New Zealand (English)
Contact your local office
Browse Course Material
Course info.
- Orhan Celiker
Departments
- Electrical Engineering and Computer Science
As Taught In
- Graphics and Visualization
- Programming Languages
Learning Resource Types
Introduction to matlab, assignments.
There are four problem sets, due daily. The first one will be released after the first class, and it will be due before the second class. The last problem set will be due before the last class. Problem set grading will be done coarsely (i.e. we will not penalize you for minor mistakes).

You are leaving MIT OpenCourseWare

IMAGES
VIDEO
COMMENTS
One area in which MATLAB excels is matrix computation. Creating a matrix is as easy as making a vector, using semicolons (;) to separate the rows of a matrix. A = [1 2 0; 2 5 -1; 4 10 -1] A = 3×3 1 2 0 2 5 -1 4 10 -1 We can easily find the ...
When the indices in an assignment operation exceed the size of the matrix, Matlab, rather than giving an error, quietly expands the matrix for you. If necessary, it pads the matrix with zeros. Using this feature is somewhat inefficient, however, as Matlab must reallocate a sufficiently large chunk of contiguous memory and copy the array.
You can assign to a block within a matrix. If the right hand side is a scalar, all values within that block are assigned that value. If the right hand side is a matrix, it must have the same dimensions of the block you are assigning to. The matrix is automatically enlarged if you index outside the matrix.
matrices.dvi. last change: 6feb12. matrices in matlab. Every variable in matlab is a matrix, even constants such as 3 (which is a 1-by-1 matrix). The size of a matrix a is provided by the command size(a) which returns a two-entry row matrix containing the number of rows and of columns of a (in that order). If the matrix a appears at a place ...
The Matlab command rand(m, n) creates an m × n matrix whose entries are random real numbers between 0 and 1. Type R = rand(2, 3). Then use the up-arrow key to generate two more samples of the random matrix R. Notice how the entries in R change each time the command is executed.
0. You are just overwriting your old D matrix with a new value, i.e. [D A1]. [D A1] concatenates the D matrix (which is empty in the beginning) horizontally with A1 (which is a row matrix). answered May 14, 2017 at 21:16. m7913d.
A Matrix is a two-dimensional array of elements. In MATLAB, the matrix is created by assigning the array elements that are delimited by spaces or commas and using semicolons to mark the end of each row. Now let's have a glance at some examples to understand it better. Syntax: Example: Creating a Matrix.
A multidimensional array in MATLAB® is an array with more than two dimensions. In a matrix, the two dimensions are represented by rows and columns. Each element is defined by two subscripts, the row index and the column index. Multidimensional arrays are an extension of 2-D matrices and use additional subscripts for indexing.
One area in which MATLAB excels is matrix computation. Creating a matrix is as easy as making a vector, using semicolons (;) to separate the rows of a matrix. A = [1 2 0; 2 5 -1; 4 10 -1] A = 3×3 1 2 0 2 5 -1 4 10 -1 We can easily find the ...
This homework is designed to give you practice with more advanced and specific Matlab functionality, like advanced data structures, images, and animation. As before, the names of helpful functions are provided in bold where needed. Homework 4 Code (ZIP) This file contains: 1 .pdf file and 1 .m file.
I have a matrix A and three vectors of the same length, r, holding the indexes of the rows to assign to, c, holding the indexes of the columns to assign to, and v containing the actual values to assign. What I want to get is A(r(i),c(i))==v(i) for all i. But doing. Doesn't yield the correct result as matlab interprets it as choosing every ...
Matrix Creation and Assignment in Matlab. Ask Question Asked 6 years, 3 months ago. Modified 6 years, 3 months ago. Viewed 81 times 0 I have two matrices A fixed size, B grows iterationwise. How to create matrix B of equal size as A, but to be enlarged/manipulated according to the vector 'j'. Cases 1) Size B< Size A, 2) Size B=Size A, 3) Size B ...