
Welcome.please sign up.
By signing up or logging in, you agree to our Terms of service and confirm that you have read our Privacy Policy .
Already a member? Go to Log In
Welcome.please login.
Forgot your password
Not registered yet? Go to Sign Up
- Introduction
- Let's start
- Variables and literals
- Decide if/else
- Controlling loop
- Inline function
- Scope of variables
- Std::vector
- Std::string
- Pre-processor
- Classes and objects
- Destructors
- Initialization list
- Constructor overloading
- Array of objects
- More about functions
- Multiple inheritance
- Virtual and abstract
- Encapsulation
- Friend class and function
- Const keyword
- Dynamic memory
- Storage classes

Arrays in C++
In simple English, array means collection. In C++ also, an array is a collection of similar types of data. eg.- an array of int will contain only integers, an array of double will contain only doubles, etc.
Suppose we need to store the marks of 50 students in a class and calculate the average marks. Declaring 50 separate variables will do the job but no programmer would like to do so. And here comes the array in action.
How to declare an array
datatype array_name [ array_size ] ;
For example, take an integer array 'n' .
n[ ] is used to denote an array named 'n' .
So, n[6] means that 'n' is an array of 6 integers. Here, 6 is the size of the array i.e., there are 6 elements of array 'n'.
Giving array size i.e. 6 is necessary because the compiler needs to allocate space to that many integers. The compiler determines the size of an array by calculating the number of elements of the array.
Here 'int n[6]' will allocate space to 6 integers.
We can also declare an array by another method.
int n[ ] = { 2,3,15,8,48,13 };
In this case, we are declaring and assigning values to the array at the same time. Hence, no need to specify the array size because the compiler gets it from { 2,3,15,8,48,13 }.
Following is the pictorial view of the array.
0,1,2,3,4 and 5 are the indices. It is like these are the identities of 6 different elements of the array. Index starts from 0. So, the first element has index 0. We access the elements of an array by writing array_name[index] .
Here, n[0] is 2 n[1] is 3 n[2] is 15 n[3] is 8 n[4] is 48 n[5] is 13
Initializing an array
By writing int n[ ]={ 2,4,8 }; , we are initializing the array.
But when we declare an array like int n[3]; , we need to assign the values to it separately. Because 'int n[3];' will definitely allocate the space of 3 integers in the memory but there are no integers in that.
To assign values to the array, assign a value to each of the element of the array.
n[0] = 2; n[1] = 4; n[2] = 8;
It is just like we are declaring some variables and then assigning the values to them.
int x,y,z; x=2; y=4; z=8;
Thus, the two ways of initializing an array are:
int n[ ]={ 2,4,8 };
and the second method is declaring the array first and then assigning the values to its elements.
int n[3]; n[0] = 2; n[1] = 4; n[2] = 8;
You can understand this by treating n[0], n[1] and n[2] as different variables you used before.
Just like a variable, an array can be of any other data type also.
float f[ ]= { 1.1, 1.4, 1.5};
Here, f is an array of floats.
First, let's see the example to calculate the average of the marks of 3 students. Here, marks[0], marks[1] and marks[2] represent the marks of the first, second and third student respectively.
Here, you have seen a working example of array. We treated the array in the exact similar way as we had treated normal variables.
We can also use for loop as in the next example.
The above code was just to make you familiar with using loops with an array because you will be doing this many times later.
The code is simple, i and j starts from 0 because index of an array starts from 0 and goes up to 9 ( for 10 elements ). So, i and j goes up to 9 and not 10 ( i<10 and j<10 ) . So in the above code, n[i] will be n[0], n[1], n[2], ...., n[9].
There are two for loops in the above example. In the first for loop, we are taking the values of the different elements of the array from the user one by one. In the second for loop, we are printing the values of the elements of the array.
Let's go to the first for loop. In the first iteration, the value of i is 0, so 'n[i]' is 'n[0]'.Thus by writing cin >> n[i]; , the user will be asked to enter the value of n[0]. Similary in the second iteration, the value of 'i' will be 1 and 'n[i]' will be 'n[1]'. So 'cin >> n[i];' will be used to input the value from the user for n[1] and so on. 'i' will go up to 9, and so indices of the array ( 0,1,2,...,9).
Suppose we initialize an array as
int n[5] = { 12, 13, 5 };
This means that n[0]=12, n[1]=13 and n[2]=5 and rest all elements are zero i.e. n[3]=0 and n[4]=0.
int n[5]; n[0] = 12; n[1] = 13; n[2] = 5;
In the above code, n[0], n[1] and n[2] are assigned values 12, 13 and 5 respectively. Therefore, n[4] and n[5] are both 0.
Pointer to Arrays
Till now, you have seen how to declare and assign values to an array. Now, you will see how we can have pointers to arrays too. But before starting, we are assuming that you have gone through Pointers. If not, then first read the topic Pointers and practice some problems from the Practice section.
As we all know that pointer is a variable whose value is the address of some other variable i.e., if a variable y points to another variable x means that the value of the variable 'y' is the address of 'x'.
Similarly, if we say that a variable y points to an array n , then it means that the value of 'y' is the address of the first element of the array i.e., n[0]. So, y is the pointer to the array n.
If p is a pointer to the array age , then it means that p(or age) points to age[0].
int age[50]; int *p; p = age;
The above code assigns the address of the first element of age to p.
Now, since p points to the first element of the array age , *p is the value of the first element of the array.
So, *p is age[0], *(p+1) is age[1], *(p+2) is age[2].
Similarly, *age is age[0] ( value at age ), *(age+1) is age[1] ( value at age+1 ), *(age+2) is age[2] ( value at age+2 ) and so on.
That's all in pointer to arrays.
Now let's see some examples.
Since p is pointing to the first element of array, so, *p or *(p+0) represents the value at p[0] or the value at the first element of p . Similarly, *(p+1) represents value at p[1] . So *(p+3) and *(p+4) represent the values at p[3] and p[4] respectively. So, accordingly, we will get the output.
The above example sums up the above concepts. Now, let's print the address of the array and also individual elements of the array.
In the above example, we saw that the address of the first element of n and p is the same. We also printed the values of other elements of the array by using (p+1), (p+2) and (p+3).
Passing the whole Array in Function
In C++, we can pass an element of an array or the full array as an argument to a function.
Let's first pass a single array element to a function.
Passing an entire Array in a Function
We can also pass a whole array to a function by passing the array name as argument. Yes, the trick is that we will pass the address of array, that is the address of the first element of the array. Thus, by having the pointer of the first element, we can get the entire array as we have done in the above examples.

Let's see an example to understand this.
average(float a[]) - It is the function that is taking an array of float. And rest of the body of the function is performing accordingly.
b = average(n) - One thing you should note here is that we passed n . And as discussed earlier, n is the pointer to the first element or pointer to the array n[] . So, we have actually passed the pointer.
In the above example in which we calculated the average of the values of the elements of an array, we already knew the size of the array i.e., 8.
Suppose, we are taking the size of the array from the user. In that case, the size of the array is not fixed. Here, we need to pass the size of the array as the second argument to the function.
The code is similar to the previous one except that we passed the size of array explicitly - float average(float a[], int size) .
We can also pass an array to a function using pointers. Let's see how.
In the above example, the address of the array i.e., address of n[0] is passed to the formal parameters of the function.
void display(int *p) - This means that the function 'display' is taking a pointer of an integer and not returning any value.
Now, we passed the pointer of an integer i.e., pointer of array n[] - 'n' as per the demand of our function 'display'. Since p is the address of the array n[] in the function 'display', i.e., the address of the first element of the array (n[0]), therefore *p represents the value of n[0]. In the for loop in the function, p++ increases the value of p by 1. So when i=0, the value of *p gets printed. Then p++ increases *p to *(p+1) and thus in the second loop, the value of *(p+1) i.e. n[1] gets printed. This loop continues till i=7 when the value of *(p+7) i.e. n[7] gets printed.

For-each loop
There is a new form of for loop which makes iterating over arrays easier. It is called for-each loop. It is used to iterate over an array. Let's see an example of this.
This is very simple. Here, the variable m will go to every element of the array ar and will take its value. So, in the first iteration, m is the 1 st element of array ar i.e. 1. In second iteration, it is the 2 nd element i.e. 2 and so on. Just focus on the syntax of this for loop, rest of the part is very easy.
What if arrays are 2 dimensional?
Yes, 2-dimensional arrays also exist and are generally known as matrix . These consist of rows and columns.
Before going into its application, let's first see how to declare and initialize a 2 D array.
Similar to one-dimensional array, we define 2-dimensional array as below.
int a[2][4];
Here, a is a 2-D array of type int which consists of 2 rows and 4 columns .
Now let's see how to initialize a 2-dimensional array.
Initialization of 2 D Array
Same as in one-dimensional array, we can assign values to a 2-dimensional array in 2 ways as well.
In the first method, just assign a value to the elements of the array. If no value is assigned to any element, then its value is assigned zero by default.
Suppose we declared a 2-dimensional array a[2][2] . Then to assign it values, we need to assign a value to its elements.
int a[2][2]; a[0][0]=1; a[0][1]=2; a[1][0]=3; a[1][1]=4;
The second way is to declare and assign values at the same time as we did in one-dimensional array.
int a[2][3] = { 1, 2, 3, 4, 5, 6 };
Here, value of a[0][0] is 1, a[0][1] is 2, a[0][2] is 3, a[1][0] is 4, a[1][1] is 5 and a[1][2] is 6.
We can also write the above code as:
int a[2][3] = { {1, 2, 3}, {4, 5, 6 } };
Let's consider different cases of initializing an array.
int a[2][2] = { 1, 2, 3, 4 }; /* valid */ int a[ ][2] = { 1, 2, 3, 4 }; /* valid */ int a[2][ ] = { 1, 2, 3, 4 }; /* invalid */ int a[ ][ ] = { 1, 2, 3, 4 }; /* invalid */
Why use 2 D Array?
Suppose we have 3 students each studying 2 subjects (subject 1 and subject 2) and we have to display the marks in both the subjects of the 3 students. Let's input the marks from the user.
In the above example, firstly we defined our array consisting of 3 rows and 2 columns as float marks[3][2];
Here, the elements of the array will contain the marks of the 3 students in the 2 subjects as follows.
In our example, firstly we are taking the value of each element of the array using a for loop inside another for loop.
In the first iteration of the outer for loop, value of 'i' is 0. With the value of 'i' as 0, when the inner for loop first iterates, the value of 'j' becomes zero and thus marks[i][j] becomes marks[0][0]. By writing cin >> marks[i][j]; , we are taking the value of marks[0][0].
After that, the inner for loop again iterates and the value of 'j' becomes 1. marks[i][j] becomes marks[0][1] and its value is taken from the user.
Then, the outer loop iterates for the second time and the value of 'i' becomes 1 and the whole process continues.
After assigning the values to the elements of the array, we are printing the values of the elements of the array, in the same way, using another for loop inside for loop.
Let's see one more example of 2 D Array
Suppose there are 2 factories and each of these factories produces items of 4 different types like some items of type 1, some items of type 2 and so on. We have to calculate the total product of each factory i.e. sum of the items of each type that a factory produces.
Here, s[0][i] represents the number of items of the first factory and type i, where i takes value from 0 to 3 using for loop and s[1][i] represents the nunmber of items of the second factory of type i. E.g. - s[0][2] represents the third type of item of first factory and s[1][2] represents the third type of item of second factory. sum1 is the sum of all these items of factory 1. Similar is the case of the second factory.
So, initally, sum1 is 0. Now in first iteration, s[0][i] is s[0][0]. This means that it will represent number of first item of first factory. So, sum1 += s[0][i] will become sum1 += s[0][0] . So, sum1 will become 2. Similarly in second iteration, s[0][i] will become s[0][1] and will represent the second type of items of first factory. Now, sum1 will become 2+5 i.e. 7 . Similarly things will go further.
You practice and you get better. It's very simple. -Phillip Glass
- Making a stack using an array in C
- Making a queue using an array in C
- Sorting an array using bubble sort in C
- Sorting an array using selection sort in C
- Sorting an array using insertion sort in C
- Generating permutations of all elements of an array
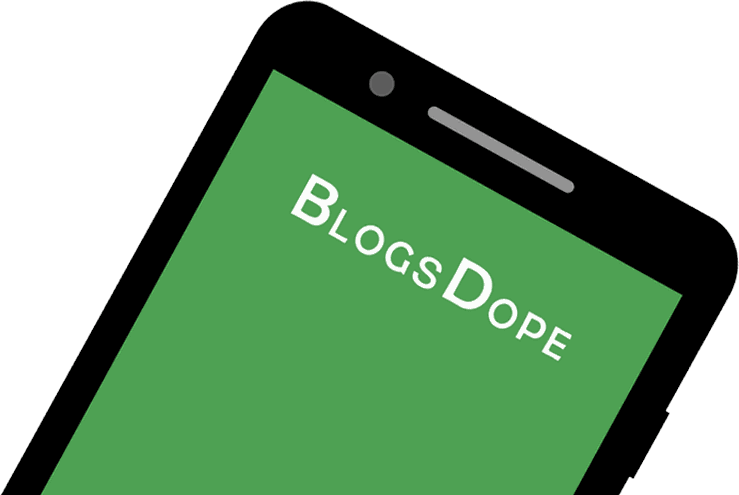
Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, introduction to c++.
- Getting Started With C++
- Your First C++ Program
- C++ Comments
C++ Fundamentals
- C++ Keywords and Identifiers
- C++ Variables, Literals and Constants
- C++ Data Types
- C++ Type Modifiers
- C++ Constants
- C++ Basic Input/Output
- C++ Operators
Flow Control
- C++ Relational and Logical Operators
- C++ if, if...else and Nested if...else
- C++ for Loop
- C++ while and do...while Loop
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ switch..case Statement
- C++ Ternary Operator
- C++ Functions
- C++ Programming Default Arguments
- C++ Function Overloading
- C++ Inline Functions
- C++ Recursion
Arrays and Strings
- C++ Array to Function
C++ Multidimensional Arrays
- C++ String Class
Pointers and References
- C++ Pointers
C++ Pointers and Arrays
- C++ References: Using Pointers
- C++ Call by Reference: Using pointers
- C++ Memory Management: new and delete
Structures and Enumerations
- C++ Structures
- C++ Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
Object Oriented Programming
- C++ Classes and Objects
- C++ Constructors
- C++ Constructor Overloading
- C++ Destructors
- C++ Access Modifiers
- C++ Encapsulation
- C++ friend Function and friend Classes
Inheritance & Polymorphism
- C++ Inheritance
- C++ Public, Protected and Private Inheritance
- C++ Multiple, Multilevel and Hierarchical Inheritance
- C++ Function Overriding
- C++ Virtual Functions
- C++ Abstract Class and Pure Virtual Function
STL - Vector, Queue & Stack
- C++ Standard Template Library
- C++ STL Containers
C++ std::array
- C++ Vectors
- C++ Forward List
- C++ Priority Queue
STL - Map & Set
- C++ Multimap
- C++ Multiset
- C++ Unordered Map
- C++ Unordered Set
- C++ Unordered Multiset
- C++ Unordered Multimap
STL - Iterators & Algorithms
- C++ Iterators
- C++ Algorithm
- C++ Functor
Additional Topics
- C++ Exceptions Handling
- C++ File Handling
- C++ Ranged for Loop
- C++ Nested Loop
- C++ Function Template
- C++ Class Templates
- C++ Type Conversion
- C++ Type Conversion Operators
- C++ Operator Overloading
Advanced Topics
- C++ Namespaces
- C++ Preprocessors and Macros
- C++ Storage Class
- C++ Bitwise Operators
- C++ Buffers
- C++ istream
- C++ ostream
C++ Tutorials
- Find Largest Element of an Array
- Access Elements of an Array Using Pointer
Passing Array to a Function in C++ Programming
- Calculate Average of Numbers Using Arrays
In C++, std::array is a container class that encapsulates fixed size arrays. It is similar to the C-style arrays as it stores multiple values of similar type.
- C++ std::array Declaration
std::array is defined in the <array> header so we must include this header before we can use std::array .
- T - Type of element to be stored
- N - Number of elements in the array
Here is how we declare a std::array of size 5 that stores a list of numbers:
- Initialization of std::array
We can initialize std::array in two ways:
Here, we initialized two arrays named numbers and marks both of size 5 that stores element of type int .
- Example: C++ std::array
In the above example, we have declared and initialized a std::array named numbers using uniform initialization.
We then displayed the content of the array using a ranged for loop .
- Accessing the Elements of std::array
We can access the element of the array using the [] operator and index of the array element.
Since the indexing starts from 0, n[0] returns the first element of the array, n[1] returns the second element and so on.
Note : Accessing elements using [] operator doesn't check for out of bound error.
An out of bounds error occurs when a program tries to access data outside the allowed range. For example, we have an array of 5 elements and we try accessing the 10th element, that's an out of bound error.
Another way to access the element of the array is to use the at method which checks for out of bound error.
- Modifying the Elements of std::array
To modify an element at a particular index, we can again use [] and at .
- Example: Modify and Access the Array Elements
The above example demonstrates the use of [] operator and the at method to access and modify the elements of std::array .
- Check if the Array is Empty
To check if the array is empty or not, we can use the empty() method as:
The empty method returns true when the array is empty and false otherwise.
- Get the Number of Elements in the Array
We can use the size method to get the number of elements in the array.
- Example: Using the empty and size method with std::array
In the above example, we used the empty method to check if the array is empty and the size method to get the number of elements in the array.
- Fill std::array With Same Value
We can use the fill method to fill the entire array with the same value.
Let's look at an example.
Here, we used a.fill(0) to fill the entire array with 0.
- Example: Using std::array with STL Algorithms
In C++, we can use the Standard Template Library to implement some of the commonly used algorithms. These algorithm supports std::array very well. For example,
Here, we performed 3 different operations in the array: sorting, searching and summing.
To sort the array, we used the sort() function.
This sorts the array from the first element to last.
To search an element in the array, we used the find() function.
Here, the find function searches if the number 5 is in the array age . The result is stored in the variable found . If found equals to age.end() , the number isn't there; otherwise, it is.
To find the sum of the elements of the array, we use the std::accumulate function.
This returns the sum of the numbers of an entire array.
The differences between std::array and C-style-array are:
- C-style array T[n] can automatically cast to T* while std::array can.
- C-style-array doesn't support assignment while std::array<T, N> does.
Moreover, std::array provides all the benefits of an STL container over a C-style array. Thus it is recommended to use std::array over C-style-array in C++.
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
C++ Tutorial
- C++ Language
- Ascii Codes
- Boolean Operations
- Numerical Bases
Introduction
Basics of c++.
- Structure of a program
- Variables and types
- Basic Input/Output
Program structure
- Statements and flow control
- Overloads and templates
- Name visibility
Compound data types
- Character sequences
- Dynamic memory
- Data structures
- Other data types
- Classes (I)
- Classes (II)
- Special members
- Friendship and inheritance
- Polymorphism
Other language features
- Type conversions
- Preprocessor directives
Standard library
- Input/output with files

Initializing arrays

Accessing the values of an array

Multidimensional arrays

Arrays as parameters
Library arrays.

11.2 — Arrays (Part II)
This lesson has been deprecated and replaced with the updated lessons in the latter half of chapter 17 (starting with lesson 17.7 -- Introduction to C-style arrays ).
This lesson continues the discussion of arrays that began in lesson 17.7 -- Introduction to C-style arrays .
Initializing fixed arrays
Array elements are treated just like normal variables, and as such, they are not initialized when created.
One way to “initialize” an array is to do it element by element:
However, this is a pain, especially as the array gets larger. Furthermore, it’s not initialization, but assignment. Assignments don’t work if the array is const .
Fortunately, C++ provides a more convenient way to initialize entire arrays via use of an initializer list . The following example initializes the array with the same values as the one above:
If there are more initializers in the list than the array can hold, the compiler will generate an error.
However, if there are less initializers in the list than the array can hold, the remaining elements are initialized to 0 (or whatever value 0 converts to for a non-integral fundamental type -- e.g. 0.0 for double). This is called zero initialization .
The following example shows this in action:
This prints:
Consequently, to initialize all the elements of an array to 0, you can do this:
If the initializer list is omitted, the elements are uninitialized, unless they are a class-type that self-initializes.
Best practice
Explicitly initialize your arrays (even when the element type is self-initializing).
Omitted length
If you are initializing a fixed array of elements using an initializer list, the compiler can figure out the length of the array for you, and you can omit explicitly declaring the length of the array.
The following two lines are equivalent:
This not only saves typing, it also means you don’t have to update the array length if you add or remove elements later.
Arrays and enums
One of the big documentation problems with arrays is that integer indices do not provide any information to the programmer about the meaning of the index. Consider a class of 5 students:
Who is represented by testScores[2]? It’s not clear.
This can be solved by setting up an enumeration where one enumerator maps to each of the possible array indices:
In this way, it’s much clearer what each of the array elements represents. Note that an extra enumerator named max_students has been added. This enumerator is used during the array declaration to ensure the array has the proper length (as the array length should be one greater than the largest index). This is useful both for documentation purposes, and because the array will automatically be resized if another enumerator is added:
Note that this “trick” only works if you do not change the enumerator values manually!
Arrays and enum classes
Enum classes don’t have an implicit conversion to integer, so if you try the following:
You’ll get a compiler error. This can be addressed by using a static_cast to convert the enumerator to an integer:
However, doing this is somewhat of a pain, so it might be better to use a standard enum inside of a namespace:
Passing arrays to functions
Although passing an array to a function at first glance looks just like passing a normal variable, underneath the hood, C++ treats arrays differently.
When a normal variable is passed by value, C++ copies the value of the argument into the function parameter. Because the parameter is a copy, changing the value of the parameter does not change the value of the original argument.
However, because copying large arrays can be very expensive, C++ does not copy an array when an array is passed into a function. Instead, the actual array is passed. This has the side effect of allowing functions to directly change the value of array elements!
The following example illustrates this concept:
In the above example, value is not changed in main() because the parameter value in function passValue() was a copy of variable value in function main(), not the actual variable. However, because the parameter array in function passArray() is the actual array, passArray() is able to directly change the value of the elements!
Why this happens is related to the way arrays are implemented in C++, a topic we’ll revisit in lesson 17.8 -- C-style array decay . For now, you can consider this as a quirk of the language.
As a side note, if you want to ensure a function does not modify the array elements passed into it, you can make the array const:
Determining the length of an array
The std::size() function from the <iterator> header can be used to determine the length of arrays.
Here’s an example:
Note that due to the way C++ passes arrays to functions, this will not work for arrays that have been passed to functions!
std::size() will work with other kinds of objects (such as std::array and std::vector), and it will cause a compiler error if you try to use it on a fixed array that has been passed to a function! Note that std::size returns an unsigned value. If you need a signed value, you can either cast the result or, since C++20, use std::ssize() (stands for signed size).
std::size() was added in C++17. If you’re using C++11 or C++14, you can use this function instead:
In older code, you may see the length calculated using the sizeof operator instead. sizeof isn’t as easy to use as std::size() and there are a few things you have to watch out for.
The sizeof operator can be used on arrays, and it will return the total size of the array (array length multiplied by element size).
On a machine with 4 byte integers and 8 byte pointers, this printed:
(You may get a different result if the size of your types are different).
One neat trick: we can determine the length of a fixed array by dividing the size of the entire array by the size of an array element:
This printed
How does this work? First, note that the size of the entire array is equal to the array’s length multiplied by the size of an element. Put more compactly: array size = array length * element size.
Using algebra, we can rearrange this equation: array length = array size / element size. sizeof(array) is the array size, and sizeof(array[0]) is the element size, so our equation becomes array length = sizeof(array) / sizeof(array[0]). Sometimes *array is used instead of array[0] (we discuss what *array is in 17.9 -- Pointer arithmetic and subscripting ).
Note that this will only work if the array is a fixed-length array, and you’re doing this trick in the same function that array is declared in (we’ll talk more about why this restriction exists in a future lesson in this chapter).
When sizeof is used on an array that has been passed to a function, it doesn’t error out like std::size() does. Instead, it returns the size of a pointer.
Again assuming 8 byte pointers and 4 byte integers, this prints
Author’s note
A properly configured compiler should print a warning if you try to use sizeof() on an array that was passed to a function.
The calculation in main() was correct, but the sizeof() in printSize() returned 8 (the size of a pointer), and 8 divided by 4 is 2.
For this reason, be careful about using sizeof() on arrays!
Note: In common usage, the terms “array size” and “array length” are both most often used to refer to the array’s length (the size of the array isn’t useful in most cases, outside of the trick we’ve shown you above).
Indexing an array out of range
Remember that an array of length N has array elements 0 through N-1. So what happens if you try to access an array with a subscript outside of that range?
Consider the following program:
In this program, our array is of length 5, but we’re trying to write a prime number into the 6th element (index 5).
C++ does not do any checking to make sure that your indices are valid for the length of your array. So in the above example, the value of 13 will be inserted into memory where the 6th element would have been had it existed. When this happens, you will get undefined behavior -- for example, this could overwrite the value of another variable, or cause your program to crash.
Although it happens less often, C++ will also let you use a negative index, with similarly undesirable results.
When using arrays, ensure that your indices are valid for the range of your array!
- Declare an array to hold the high temperature (to the nearest tenth of a degree) for each day of a year (assume 365 days in a year). Initialize the array with a value of 0.0 for each day.
- Set up an enum with the names of the following animals: chicken, dog, cat, elephant, duck, and snake. Put the enum in a namespace. Define an array with an element for each of these animals, and use an initializer list to initialize each element to hold the number of legs that animal has.
Write a main function that prints the number of legs an elephant has, using the enumerator.
Quiz answers
- Show Solution
C++ Tutorial
C++ functions, c++ classes, c++ reference, c++ examples.
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value.
To declare an array, define the variable type, specify the name of the array followed by square brackets and specify the number of elements it should store:
We have now declared a variable that holds an array of four strings. To insert values to it, we can use an array literal - place the values in a comma-separated list, inside curly braces:
To create an array of three integers, you could write:
Access the Elements of an Array
You access an array element by referring to the index number inside square brackets [] .
This statement accesses the value of the first element in cars :
Note: Array indexes start with 0: [0] is the first element. [1] is the second element, etc.
Change an Array Element
To change the value of a specific element, refer to the index number:
C++ Exercises
Test yourself with exercises.
Create an array of type string called cars .
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
array Class (C++ Standard Library)
- 11 contributors
Describes an object that controls a sequence of length N of elements of type Ty . The sequence is stored as an array of Ty , contained in the array<Ty, N> object.
Ty The type of an element.
N The number of elements.
The type has a default constructor array() and a default assignment operator operator= , and satisfies the requirements for an aggregate . Therefore, objects of type array<Ty, N> can be initialized by using an aggregate initializer. For example,
creates the object ai that holds four integer values, initializes the first three elements to the values 1, 2, and 3, respectively, and initializes the fourth element to 0.
Requirements
Header: <array>
Namespace: std
array::array
Constructs an array object.
right Object or range to insert.
The default constructor array() leaves the controlled sequence uninitialized (or default initialized). You use it to specify an uninitialized controlled sequence.
The copy constructor array(const array& right) initializes the controlled sequence with the sequence [ right .begin() , right .end() ). You use it to specify an initial controlled sequence that is a copy of the sequence controlled by the array object right .

array::assign
Obsolete in C++11, replaced by fill . Replaces all elements.
Accesses an element at a specified position.
off Position of element to access.
The member functions return a reference to the element of the controlled sequence at position off . If that position is invalid, the function throws an object of class out_of_range .
array::back
Accesses the last element.
The member functions return a reference to the last element of the controlled sequence, which must be non-empty.
array::begin
Designates the beginning of the controlled sequence.
The member functions return a random-access iterator that points at the first element of the sequence (or just beyond the end of an empty sequence).
array::cbegin
Returns a const iterator that addresses the first element in the range.
Return Value
A const random-access iterator that points at the first element of the range, or the location just beyond the end of an empty range (for an empty range, cbegin() == cend() ).
With the return value of cbegin , the elements in the range can't be modified.
You can use this member function in place of the begin() member function to guarantee that the return value is const_iterator . Typically, it's used with the auto type deduction keyword, as shown in the following example. In the example, consider Container to be a modifiable (non- const ) container of any kind that supports begin() and cbegin() .
array::cend
Returns a const iterator that addresses the location just beyond the last element in a range.
A random-access iterator that points just beyond the end of the range.
cend is used to test whether an iterator has passed the end of its range.
You can use this member function in place of the end() member function to guarantee that the return value is const_iterator . Typically, it's used with the auto type deduction keyword, as shown in the following example. In the example, consider Container to be a modifiable (non- const ) container of any kind that supports end() and cend() .
The value returned by cend shouldn't be dereferenced.
array::const_iterator
The type of a constant iterator for the controlled sequence.
The type describes an object that can serve as a constant random-access iterator for the controlled sequence.
array::const_pointer
The type of a constant pointer to an element.
The type describes an object that can serve as a constant pointer to elements of the sequence.
array::const_reference
The type of a constant reference to an element.
The type describes an object that can serve as a constant reference to an element of the controlled sequence.
array::const_reverse_iterator
The type of a constant reverse iterator for the controlled sequence.
The type describes an object that can serve as a constant reverse iterator for the controlled sequence.
array::crbegin
Returns a const iterator to the first element in a reversed array.
A const reverse random-access iterator addressing the first element in a reversed array or addressing what had been the last element in the unreversed array.
With the return value of crbegin , the array object can't be modified.
array::crend
Returns a const iterator that addresses the location succeeding the last element in a reversed array.
A const reverse random-access iterator that addresses the location succeeding the last element in a reversed array (the location that had preceded the first element in the unreversed array).
crend is used with a reversed array just as array::cend is used with an array.
With the return value of crend (suitably decremented), the array object can't be modified.
crend can be used to test to whether a reverse iterator has reached the end of its array.
The value returned by crend shouldn't be dereferenced.
array::data
Gets the address of the first element.
The member functions return the address of the first element in the controlled sequence.
array::difference_type
The type of a signed distance between two elements.
The signed integer type describes an object that can represent the difference between the addresses of any two elements in the controlled sequence. It's a synonym for the type std::ptrdiff_t .
array::empty
Tests whether no elements are present.
The member function returns true only if N == 0 .
Designates the end of the controlled sequence.
The member functions return a random-access iterator that points just beyond the end of the sequence.
array::fill
Erases an array and copies the specified elements to the empty array.
val The value of the element being inserted into the array.
fill replaces each element of the array with the specified value.
array::front
Accesses the first element.
The member functions return a reference to the first element of the controlled sequence, which must be non-empty.
array::iterator
The type of an iterator for the controlled sequence.
The type describes an object that can serve as a random-access iterator for the controlled sequence.
array::max_size
Counts the number of elements.
The member function returns N .
array::operator[]
The member functions return a reference to the element of the controlled sequence at position off . If that position is invalid, the behavior is undefined.
There's also a non-member get function available to get a reference to an element of an array .
array::operator=
Replaces the controlled sequence.
right Container to copy.
The member operator assigns each element of right to the corresponding element of the controlled sequence, then returns *this . You use it to replace the controlled sequence with a copy of the controlled sequence in right .
array::pointer
The type of a pointer to an element.
The type describes an object that can serve as a pointer to elements of the sequence.
array::rbegin
Designates the beginning of the reversed controlled sequence.
The member functions return a reverse iterator that points just beyond the end of the controlled sequence. Hence, it designates the beginning of the reverse sequence.
array::reference
The type of a reference to an element.
The type describes an object that can serve as a reference to an element of the controlled sequence.
array::rend
Designates the end of the reversed controlled sequence.
The member functions return a reverse iterator that points at the first element of the sequence (or just beyond the end of an empty sequence)). Hence, it designates the end of the reverse sequence.
array::reverse_iterator
The type of a reverse iterator for the controlled sequence.
The type describes an object that can serve as a reverse iterator for the controlled sequence.
array::size
Array::size_type.
The type of an unsigned distance between two elements.
The unsigned integer type describes an object that can represent the length of any controlled sequence. It's a synonym for the type std::size_t .
array::swap
Swaps the contents of this array with another array.
right Array to swap contents with.
The member function swaps the controlled sequences between *this and right . It performs element assignments and constructor calls proportional to N .
There's also a non-member swap function available to swap two array instances.
array::value_type
The type of an element.
The type is a synonym for the template parameter Ty .
<array>
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- Operator Precedence and Associativity in C
- Difference between Operator Precedence and Operator Associativity
- Operator Associativity in Programming
- Operator precedence in JavaScript
- Floating Point Operations & Associativity in C, C++ and Java
- Precedence of postfix ++ and prefix ++ in C/C++
- Bitwise AND operator in Programming
- Assignment Operators In C++
- Assignment Operators in C
- Result of comma operator as l-value in C and C++
- Self assignment check in assignment operator
- Assignment Operators in Programming
- bitset operator[] in C++ STL
- Precedence and Associativity of Operators in Python
- Operator grammar and precedence parser in TOC
- Operator Precedence in Ruby
- MySQL | Operator precedence
- Operators Precedence in Scala
- Precedence Graph in Operating System
Operator Precedence and Associativity in C++
In C++, operator precedence and associativity are important concepts that determine the order in which operators are evaluated in an expression. Operator precedence tells the priority of operators, while associativity determines the order of evaluation when multiple operators of the same precedence level are present.
Operator Precedence in C++
In C++, operator precedence specifies the order in which operations are performed within an expression. When an expression contains multiple operators, those with higher precedence are evaluated before those with lower precedence.
For expression:
As, multiplication has higher precedence than subtraction, that’s why 17 * 6 is evaluated first, resulting in x = -97 . If we want to evaluate 5 – 17 first, we can use parentheses:
Now 5 – 17 is evaluated first, resulting in x = -72 .
Example of C++ Operator Precedence
Remember that, using parentheses makes code more readable, especially when dealing with complex expressions.
Operator Associativity in C++
Operator associativity determines the order in which operands are grouped when multiple operators have the same precedence. There are two types of associativity:
Left-to-right associativity: In expressions like a + b – c, the addition and subtraction operators are evaluated from left to right. So, (a + b) – c is equivalent.
Right-to-left associativity: Some operators, like the assignment operator =, have right-to-left associativity. For example, a = b = 4; assigns the value of b to a.
As, subtraction is left-associative, that’s why 10 – 5 evaluated first, resulting in x = 3 . But in case of multiplication:
Now 3 * 4 is evaluated first, resulting in x = 24 because multiplication is right-associative.
Example of C++ Operator Associativity
Understanding both the precedence and associativity of operators is crucial for writing expressions that produce the desired results.
Operator Precedence Table in C++
The operator precedence table in C++ is a table that lists the operators in order of their precedence level. Operators with higher precedence are evaluated before operators with lower precedence. This table also includes the associativity of the operators, which determines the order in which operators of the same precedence are processed.
The operators are listed from top to bottom, in descending precedence
Please Login to comment...
Similar reads.
- cpp-operator
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
cppreference.com
Assignment operators.
Assignment operators modify the value of the object.
[ edit ] Definitions
Copy assignment replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is performed in a special member function, described in copy assignment operator .
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
Compound assignment replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
[ edit ] Assignment operator syntax
The assignment expressions have the form
- ↑ target-expr must have higher precedence than an assignment expression.
- ↑ new-value cannot be a comma expression, because its precedence is lower.
[ edit ] Built-in simple assignment operator
For the built-in simple assignment, the object referred to by target-expr is modified by replacing its value with the result of new-value . target-expr must be a modifiable lvalue.
The result of a built-in simple assignment is an lvalue of the type of target-expr , referring to target-expr . If target-expr is a bit-field , the result is also a bit-field.
[ edit ] Assignment from an expression
If new-value is an expression, it is implicitly converted to the cv-unqualified type of target-expr . When target-expr is a bit-field that cannot represent the value of the expression, the resulting value of the bit-field is implementation-defined.
If target-expr and new-value identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same).
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
[ edit ] Built-in compound assignment operator
The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the behavior of the expression target-expr = target-expr op new-value , except that target-expr is evaluated only once.
The requirements on target-expr and new-value of built-in simple assignment operators also apply. Furthermore:
- For + = and - = , the type of target-expr must be an arithmetic type or a pointer to a (possibly cv-qualified) completely-defined object type .
- For all other compound assignment operators, the type of target-expr must be an arithmetic type.
In overload resolution against user-defined operators , for every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2 , where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
Operator precedence
Operator overloading
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 January 2024, at 23:41.
- This page has been accessed 426,618 times.
- Privacy policy
- About cppreference.com
- Disclaimers


IMAGES
VIDEO
COMMENTS
Properties of Arrays in C++. An Array is a collection of data of the same data type, stored at a contiguous memory location. Indexing of an array starts from 0. It means the first element is stored at the 0th index, the second at 1st, and so on. Elements of an array can be accessed using their indices.
85. There is a difference between initialization and assignment. What you want to do is not initialization, but assignment. But such assignment to array is not possible in C++. Here is what you can do: #include <algorithm>. int array [] = {1,3,34,5,6}; int newarr [] = {34,2,4,5,6}; std::ranges::copy(newarr, array); // C++20.
C++ Arrays. In C++, an array is a variable that can store multiple values of the same type. For example, Suppose a class has 27 students, and we need to store all their grades. Instead of creating 27 separate variables, we can simply create an array: double grade[27]; Here, grade is an array that can hold a maximum of 27 elements of double type.
A declaration of the form T a [N];, declares a as an array object that consists of N contiguously allocated objects of type T.The elements of an array are numbered 0 , …, N -1, and may be accessed with the subscript operator [], as in a [0], …, a [N -1].. Arrays can be constructed from any fundamental type (except void), pointers, pointers to members, classes, enumerations, or from other ...
Here, n[0] is 2 n[1] is 3 n[2] is 15 n[3] is 8 n[4] is 48 n[5] is 13 Initializing an array. By writing int n[ ]={ 2,4,8 };, we are initializing the array.. But when we declare an array like int n[3];, we need to assign the values to it separately.Because 'int n[3];' will definitely allocate the space of 3 integers in the memory but there are no integers in that.
C++ std::array Declaration. std::array is defined in the <array> header so we must include this header before we can use std::array.. Syntax. #include <array> // declaration of std::array std::array<T, N> array_name; where, T - Type of element to be stored; N - Number of elements in the array; Here is how we declare a std::array of size 5 that stores a list of numbers:
NOTE: The elements field within square brackets [], representing the number of elements in the array, must be a constant expression, since arrays are blocks of static memory whose size must be determined at compile time, before the program runs. Initializing arrays By default, regular arrays of local scope (for example, those declared within a function) are left uninitialized.
Show 7 more. An array is a sequence of objects of the same type that occupy a contiguous area of memory. Traditional C-style arrays are the source of many bugs, but are still common, especially in older code bases. In modern C++, we strongly recommend using std::vector or std::array instead of C-style arrays described in this section.
Arrays allocate their elements contiguously in memory, and allow fast, direct access to any element via subscripting. C++ has three different array types that are commonly used: std::vector, std::array, and C-style arrays. In lesson 16.10 -- std::vector resizing and capacity, we mentioned that arrays fall into two categories:
std::array is a container that encapsulates fixed size arrays.. This container is an aggregate type with the same semantics as a struct holding a C-style array T [N] as its only non-static data member. Unlike a C-style array, it doesn't decay to T * automatically. As an aggregate type, it can be initialized with aggregate-initialization given at most N initializers that are convertible to T ...
Furthermore, it's not initialization, but assignment. Assignments don't work if the array is const. Fortunately, C++ provides a more convenient way to initialize entire arrays via use of an initializer list. The following example initializes the array with the same values as the one above: ... Declare an array to hold the high temperature ...
Variable-length arrays. If expression is not an integer constant expression, the declarator is for an array of variable size.. Each time the flow of control passes over the declaration, expression is evaluated (and it must always evaluate to a value greater than zero), and the array is allocated (correspondingly, lifetime of a VLA ends when the declaration goes out of scope).
C++ Arrays. Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To declare an array, define the variable type, specify the name of the array followed by square brackets and specify the number of elements it should store: string cars [4]; We have now declared a variable that ...
Remarks. The type has a default constructor array() and a default assignment operator operator=, and satisfies the requirements for an aggregate.Therefore, objects of type array<Ty, N> can be initialized by using an aggregate initializer. For example, array<int, 4> ai = { 1, 2, 3 }; creates the object ai that holds four integer values, initializes the first three elements to the values 1, 2 ...
Initialization from strings. String literal (optionally enclosed in braces) may be used as the initializer for an array of matching type: . ordinary string literals and UTF-8 string literals (since C11) can initialize arrays of any character type (char, signed char, unsigned char) ; L-prefixed wide string literals can be used to initialize arrays of any type compatible with (ignoring cv ...
Vectors are same as dynamic arrays with the ability to resize itself automatically when an element is inserted or deleted, with their storage being handled automatically by the container. vector::operator= This operator is used to assign new contents to the container by replacing the existing contents. It also modifies the size according to the new
Variants. Assignment operators. Assignment operators modify the value of the object. All built-in assignment operators return *this, and most user-defined overloads also return *this so that the user-defined operators can be used in the same manner as the built-ins. However, in a user-defined operator overload, any type can be used as return ...
a = "Hi"; The first is an initialization, the second is an assignment. The first line allocates enough space on the stack to hold 10 characters, and initializes the first three of those characters to be 'H', 'i', and '\0'. From this point on, all a does is refer to the position of the the array on the stack. Because the array is just a place on ...
The declaration char hello[4096]; assigns stack space for 4096 chars, indexed from 0 to 4095. Hence, hello[4096] is invalid. While this information is true, it does not answer the question that was asked. One could replace hello[4096] = 0; with hello[4095] = 0; without changing the essence of what is being asked.