

Nick McCullum
Software Developer & Professional Explainer
NumPy Indexing and Assignment
Hey - Nick here! This page is a free excerpt from my $199 course Python for Finance, which is 50% off for the next 50 students.
If you want the full course, click here to sign up.
In this lesson, we will explore indexing and assignment in NumPy arrays.
The Array I'll Be Using In This Lesson
As before, I will be using a specific array through this lesson. This time it will be generated using the np.random.rand method. Here's how I generated the array:
Here is the actual array:
To make this array easier to look at, I will round every element of the array to 2 decimal places using NumPy's round method:
Here's the new array:
How To Return A Specific Element From A NumPy Array
We can select (and return) a specific element from a NumPy array in the same way that we could using a normal Python list: using square brackets.
An example is below:
We can also reference multiple elements of a NumPy array using the colon operator. For example, the index [2:] selects every element from index 2 onwards. The index [:3] selects every element up to and excluding index 3. The index [2:4] returns every element from index 2 to index 4, excluding index 4. The higher endpoint is always excluded.
A few example of indexing using the colon operator are below.
Element Assignment in NumPy Arrays
We can assign new values to an element of a NumPy array using the = operator, just like regular python lists. A few examples are below (note that this is all one code block, which means that the element assignments are carried forward from step to step).
arr[2:5] = 0.5
Returns array([0. , 0. , 0.5, 0.5, 0.5])
As you can see, modifying second_new_array also changed the value of new_array .
Why is this?
By default, NumPy does not create a copy of an array when you reference the original array variable using the = assignment operator. Instead, it simply points the new variable to the old variable, which allows the second variable to make modification to the original variable - even if this is not your intention.
This may seem bizarre, but it does have a logical explanation. The purpose of array referencing is to conserve computing power. When working with large data sets, you would quickly run out of RAM if you created a new array every time you wanted to work with a slice of the array.
Fortunately, there is a workaround to array referencing. You can use the copy method to explicitly copy a NumPy array.
An example of this is below.
As you can see below, making modifications to the copied array does not alter the original.
So far in the lesson, we have only explored how to reference one-dimensional NumPy arrays. We will now explore the indexing of two-dimensional arrays.
Indexing Two-Dimensional NumPy Arrays
To start, let's create a two-dimensional NumPy array named mat :
There are two ways to index a two-dimensional NumPy array:
- mat[row, col]
- mat[row][col]
I personally prefer to index using the mat[row][col] nomenclature because it is easier to visualize in a step-by-step fashion. For example:
You can also generate sub-matrices from a two-dimensional NumPy array using this notation:
Array referencing also applies to two-dimensional arrays in NumPy, so be sure to use the copy method if you want to avoid inadvertently modifying an original array after saving a slice of it into a new variable name.
Conditional Selection Using NumPy Arrays
NumPy arrays support a feature called conditional selection , which allows you to generate a new array of boolean values that state whether each element within the array satisfies a particular if statement.
An example of this is below (I also re-created our original arr variable since its been awhile since we've seen it):
You can also generate a new array of values that satisfy this condition by passing the condition into the square brackets (just like we do for indexing).
An example of this is below:
Conditional selection can become significantly more complex than this. We will explore more examples in this section's associated practice problems.
In this lesson, we explored NumPy array indexing and assignment in thorough detail. We will solidify your knowledge of these concepts further by working through a batch of practice problems in the next section.
Multiple assignment in Python: Assign multiple values or the same value to multiple variables
In Python, the = operator is used to assign values to variables.
You can assign values to multiple variables in one line.
Assign multiple values to multiple variables
Assign the same value to multiple variables.
You can assign multiple values to multiple variables by separating them with commas , .
You can assign values to more than three variables, and it is also possible to assign values of different data types to those variables.
When only one variable is on the left side, values on the right side are assigned as a tuple to that variable.
If the number of variables on the left does not match the number of values on the right, a ValueError occurs. You can assign the remaining values as a list by prefixing the variable name with * .
For more information on using * and assigning elements of a tuple and list to multiple variables, see the following article.
- Unpack a tuple and list in Python
You can also swap the values of multiple variables in the same way. See the following article for details:
- Swap values in a list or values of variables in Python
You can assign the same value to multiple variables by using = consecutively.
For example, this is useful when initializing multiple variables with the same value.
After assigning the same value, you can assign a different value to one of these variables. As described later, be cautious when assigning mutable objects such as list and dict .
You can apply the same method when assigning the same value to three or more variables.
Be careful when assigning mutable objects such as list and dict .
If you use = consecutively, the same object is assigned to all variables. Therefore, if you change the value of an element or add a new element in one variable, the changes will be reflected in the others as well.
If you want to handle mutable objects separately, you need to assign them individually.
after c = []; d = [] , c and d are guaranteed to refer to two different, unique, newly created empty lists. (Note that c = d = [] assigns the same object to both c and d .) 3. Data model — Python 3.11.3 documentation
You can also use copy() or deepcopy() from the copy module to make shallow and deep copies. See the following article.
- Shallow and deep copy in Python: copy(), deepcopy()
Related Categories
Related articles.
- NumPy: arange() and linspace() to generate evenly spaced values
- Chained comparison (a < x < b) in Python
- pandas: Get first/last n rows of DataFrame with head() and tail()
- pandas: Filter rows/columns by labels with filter()
- Get the filename, directory, extension from a path string in Python
- Sign function in Python (sign/signum/sgn, copysign)
- How to flatten a list of lists in Python
- None in Python
- Create calendar as text, HTML, list in Python
- NumPy: Insert elements, rows, and columns into an array with np.insert()
- Shuffle a list, string, tuple in Python (random.shuffle, sample)
- Add and update an item in a dictionary in Python
- Cartesian product of lists in Python (itertools.product)
- Remove a substring from a string in Python
- pandas: Extract rows that contain specific strings from a DataFrame
Multi-Dimensional Arrays in Python – Matrices Explained with Examples

Multi-dimensional arrays, also known as matrices, are a powerful data structure in Python. They allow you to store and manipulate data in multiple dimensions or axes.
You'll commonly use these types of arrays in fields such as mathematics, statistics, and computer science to represent and process structured data, such as images, videos, and scientific data.
In Python, you can create multi-dimensional arrays using various libraries, such as NumPy, Pandas, and TensorFlow. In this article, we will focus on NumPy, which is one of the most popular and widely used libraries for working with arrays in Python.
NumPy provides a powerful N-dimensional array object that you can use to create and manipulate multi-dimensional arrays efficiently. We'll now look at some examples of how to create and work with multi-dimensional arrays in Python using NumPy.
How to Create Multi-Dimensional Arrays Using NumPy
To create a multi-dimensional array using NumPy, we can use the np.array() function and pass in a nested list of values as an argument. The outer list represents the rows of the array, and the inner lists represent the columns.
Here is an example of how to create a 2-dimensional array using NumPy:
In this example, we first import the NumPy library using the import statement. Then, we create a 2-dimensional array using the np.array() function and pass in a list of lists as an argument. Each inner list represents a row of the array, and the outer list contains all the rows. Finally, we print the array using the print() function.
NumPy also provides other functions to create multi-dimensional arrays, such as np.zeros() , np.ones() , and np.random.rand() . You can use these functions to create arrays of specific shapes and sizes with default or random values.
How to Access and Modify Multi-dimensional Arrays Using NumPy
Once we have created a multi-dimensional array, we can access and modify its elements using indexing and slicing. We use the index notation [i, j] to access an element at row i and column j , where i and j are zero-based indices.
Here's an example of how to access and modify elements of a 2-dimensional array using NumPy:
In this example, we create a 2-dimensional array using the np.array() function, and then access an element at row 1, column 2 using indexing. We then modify an element at row 0, column 3 using indexing again. Finally, we print the modified array using the print() function.
We can also use slicing to access and modify multiple elements of a multi-dimensional array at once. We use the slice notation arr[i:j, k:l] to access a subarray that contains rows i through j-1 and columns k through l-1 .
Here's an example of how to use slicing to access and modify elements of a 2-dimensional array using NumPy:
In this example, we create a 2-dimensional array using the np.array() function, and then use slicing to access a subarray that contains rows 0 through 1 and columns 1 through 2. We then modify the subarray by multiplying it by 2, and print the modified original array using the print() function.
How to Perform Operations on Multi-dimensional Arrays
NumPy provides a wide range of mathematical and statistical functions that you can use to perform operations on multi-dimensional arrays efficiently. These functions can help you perform element-wise operations, matrix operations, and other operations on arrays with different shapes and sizes.
Here's an example of how to perform some common operations on a 2-dimensional array using NumPy:
In this example, we create a 2-dimensional array using the np.array() function, and then use various NumPy functions to perform operations on the array.
We first calculate the sum of all elements using the np.sum() function. We then calculate the mean of each row using the np.mean() function and specify the axis=1 parameter to calculate the mean along each row. Finally, we calculate the dot product of the 2-dimensional array and another 2-dimensional array b using the np.dot() function.
Multi-dimensional arrays are a powerful and important data structure in Python. They allow us to store and manipulate large amounts of data efficiently.
In this article, we have covered the basics of creating and manipulating multi-dimensional arrays using NumPy in Python. We have also looked at some common operations that we can perform on multi-dimensional arrays using NumPy functions.
With the knowledge gained from this article, you should now be able to create and manipulate multi-dimensional arrays to suit your specific needs in Python.
Let’s connect on Twitter and LinkedIn .
Data Scientist||Machine Learning Engineer|| Data Analyst|| Microsoft Student Learn Ambassador
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Comprehensive Learning Paths
- 150+ Hours of Videos
- Complete Access to Jupyter notebooks, Datasets, References.

101 NumPy Exercises for Data Analysis (Python)
- February 26, 2018
- Selva Prabhakaran
The goal of the numpy exercises is to serve as a reference as well as to get you to apply numpy beyond the basics. The questions are of 4 levels of difficulties with L1 being the easiest to L4 being the hardest.
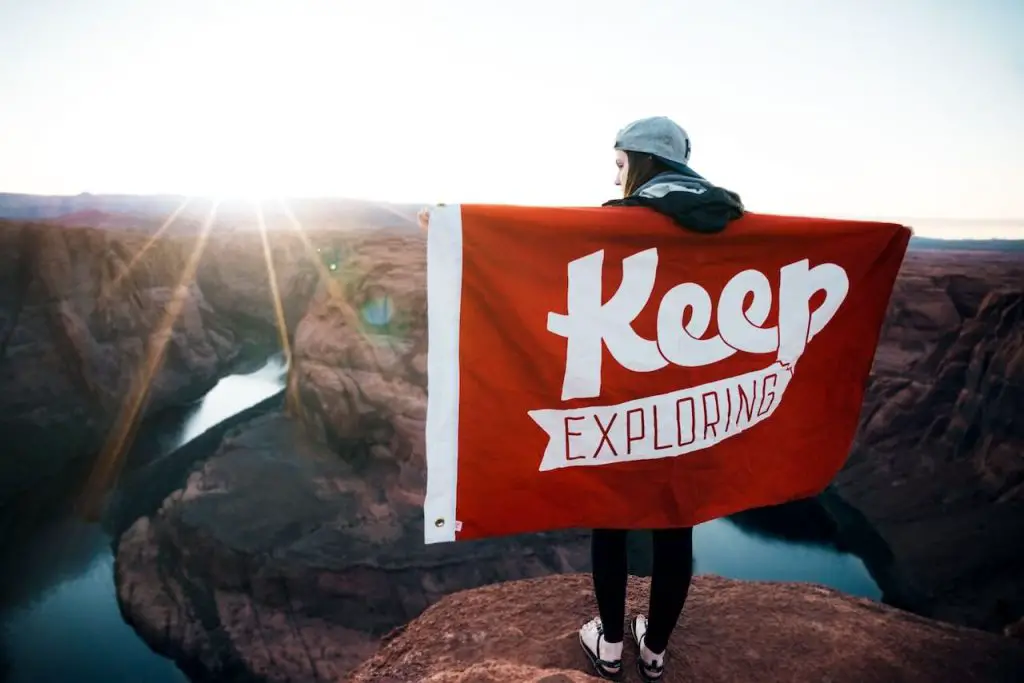
If you want a quick refresher on numpy, the following tutorial is best: Numpy Tutorial Part 1: Introduction Numpy Tutorial Part 2: Advanced numpy tutorials .
Related Post: 101 Practice exercises with pandas .
1. Import numpy as np and see the version
Difficulty Level: L1
Q. Import numpy as np and print the version number.
You must import numpy as np for the rest of the codes in this exercise to work.
To install numpy its recommended to use the installation provided by anaconda.
2. How to create a 1D array?
Q. Create a 1D array of numbers from 0 to 9
Desired output:
3. How to create a boolean array?
Q. Create a 3×3 numpy array of all True’s

4. How to extract items that satisfy a given condition from 1D array?
Q. Extract all odd numbers from arr
5. How to replace items that satisfy a condition with another value in numpy array?
Q. Replace all odd numbers in arr with -1
Desired Output:
6. How to replace items that satisfy a condition without affecting the original array?
Difficulty Level: L2
Q. Replace all odd numbers in arr with -1 without changing arr
7. How to reshape an array?
Q. Convert a 1D array to a 2D array with 2 rows
8. How to stack two arrays vertically?
Q. Stack arrays a and b vertically
9. How to stack two arrays horizontally?
Q. Stack the arrays a and b horizontally.
10. How to generate custom sequences in numpy without hardcoding?
Q. Create the following pattern without hardcoding. Use only numpy functions and the below input array a .
11. How to get the common items between two python numpy arrays?
Q. Get the common items between a and b
12. How to remove from one array those items that exist in another?
Q. From array a remove all items present in array b
13. How to get the positions where elements of two arrays match?
Q. Get the positions where elements of a and b match
14. How to extract all numbers between a given range from a numpy array?
Q. Get all items between 5 and 10 from a .
15. How to make a python function that handles scalars to work on numpy arrays?
Q. Convert the function maxx that works on two scalars, to work on two arrays.
16. How to swap two columns in a 2d numpy array?
Q. Swap columns 1 and 2 in the array arr .
17. How to swap two rows in a 2d numpy array?
Q. Swap rows 1 and 2 in the array arr :
18. How to reverse the rows of a 2D array?
Q. Reverse the rows of a 2D array arr .
19. How to reverse the columns of a 2D array?
Q. Reverse the columns of a 2D array arr .
20. How to create a 2D array containing random floats between 5 and 10?
Q. Create a 2D array of shape 5x3 to contain random decimal numbers between 5 and 10.
21. How to print only 3 decimal places in python numpy array?
Q. Print or show only 3 decimal places of the numpy array rand_arr .
22. How to pretty print a numpy array by suppressing the scientific notation (like 1e10)?
Q. Pretty print rand_arr by suppressing the scientific notation (like 1e10)
23. How to limit the number of items printed in output of numpy array?
Q. Limit the number of items printed in python numpy array a to a maximum of 6 elements.
24. How to print the full numpy array without truncating
Q. Print the full numpy array a without truncating.
25. How to import a dataset with numbers and texts keeping the text intact in python numpy?
Q. Import the iris dataset keeping the text intact.
Since we want to retain the species, a text field, I have set the dtype to object . Had I set dtype=None , a 1d array of tuples would have been returned.
26. How to extract a particular column from 1D array of tuples?
Q. Extract the text column species from the 1D iris imported in previous question.
27. How to convert a 1d array of tuples to a 2d numpy array?
Q. Convert the 1D iris to 2D array iris_2d by omitting the species text field.
28. How to compute the mean, median, standard deviation of a numpy array?
Difficulty: L1
Q. Find the mean, median, standard deviation of iris's sepallength (1st column)
29. How to normalize an array so the values range exactly between 0 and 1?
Difficulty: L2
Q. Create a normalized form of iris 's sepallength whose values range exactly between 0 and 1 so that the minimum has value 0 and maximum has value 1.
30. How to compute the softmax score?
Difficulty Level: L3
Q. Compute the softmax score of sepallength .
31. How to find the percentile scores of a numpy array?
Q. Find the 5th and 95th percentile of iris's sepallength
32. How to insert values at random positions in an array?
Q. Insert np.nan values at 20 random positions in iris_2d dataset
33. How to find the position of missing values in numpy array?
Q. Find the number and position of missing values in iris_2d 's sepallength (1st column)
34. How to filter a numpy array based on two or more conditions?
Q. Filter the rows of iris_2d that has petallength (3rd column) > 1.5 and sepallength (1st column) < 5.0
35. How to drop rows that contain a missing value from a numpy array?
Difficulty Level: L3:
Q. Select the rows of iris_2d that does not have any nan value.
36. How to find the correlation between two columns of a numpy array?
Q. Find the correlation between SepalLength(1st column) and PetalLength(3rd column) in iris_2d
37. How to find if a given array has any null values?
Q. Find out if iris_2d has any missing values.
38. How to replace all missing values with 0 in a numpy array?
Q. Replace all ccurrences of nan with 0 in numpy array
39. How to find the count of unique values in a numpy array?
Q. Find the unique values and the count of unique values in iris's species
40. How to convert a numeric to a categorical (text) array?
Q. Bin the petal length (3rd) column of iris_2d to form a text array, such that if petal length is:
- Less than 3 --> 'small'
- 3-5 --> 'medium'
- '>=5 --> 'large'
41. How to create a new column from existing columns of a numpy array?
Q. Create a new column for volume in iris_2d, where volume is (pi x petallength x sepal_length^2)/3
42. How to do probabilistic sampling in numpy?
Q. Randomly sample iris 's species such that setose is twice the number of versicolor and virginica
Approach 2 is preferred because it creates an index variable that can be used to sample 2d tabular data.
43. How to get the second largest value of an array when grouped by another array?
Q. What is the value of second longest petallength of species setosa
44. How to sort a 2D array by a column
Q. Sort the iris dataset based on sepallength column.
45. How to find the most frequent value in a numpy array?
Q. Find the most frequent value of petal length (3rd column) in iris dataset.
46. How to find the position of the first occurrence of a value greater than a given value?
Q. Find the position of the first occurrence of a value greater than 1.0 in petalwidth 4th column of iris dataset.
47. How to replace all values greater than a given value to a given cutoff?
Q. From the array a , replace all values greater than 30 to 30 and less than 10 to 10.
48. How to get the positions of top n values from a numpy array?
Q. Get the positions of top 5 maximum values in a given array a .

49. How to compute the row wise counts of all possible values in an array?
Difficulty Level: L4
Q. Compute the counts of unique values row-wise.
Output contains 10 columns representing numbers from 1 to 10. The values are the counts of the numbers in the respective rows. For example, Cell(0,2) has the value 2, which means, the number 3 occurs exactly 2 times in the 1st row.
50. How to convert an array of arrays into a flat 1d array?
Difficulty Level: 2
Q. Convert array_of_arrays into a flat linear 1d array.
51. How to generate one-hot encodings for an array in numpy?
Difficulty Level L4
Q. Compute the one-hot encodings (dummy binary variables for each unique value in the array)
52. How to create row numbers grouped by a categorical variable?
Q. Create row numbers grouped by a categorical variable. Use the following sample from iris species as input.
53. How to create groud ids based on a given categorical variable?
Q. Create group ids based on a given categorical variable. Use the following sample from iris species as input.
54. How to rank items in an array using numpy?
Q. Create the ranks for the given numeric array a .
55. How to rank items in a multidimensional array using numpy?
Q. Create a rank array of the same shape as a given numeric array a .
56. How to find the maximum value in each row of a numpy array 2d?
DifficultyLevel: L2
Q. Compute the maximum for each row in the given array.
57. How to compute the min-by-max for each row for a numpy array 2d?
DifficultyLevel: L3
Q. Compute the min-by-max for each row for given 2d numpy array.
58. How to find the duplicate records in a numpy array?
Q. Find the duplicate entries (2nd occurrence onwards) in the given numpy array and mark them as True . First time occurrences should be False .
59. How to find the grouped mean in numpy?
Difficulty Level L3
Q. Find the mean of a numeric column grouped by a categorical column in a 2D numpy array
Desired Solution:
60. How to convert a PIL image to numpy array?
Q. Import the image from the following URL and convert it to a numpy array.
URL = 'https://upload.wikimedia.org/wikipedia/commons/8/8b/Denali_Mt_McKinley.jpg'
61. How to drop all missing values from a numpy array?
Q. Drop all nan values from a 1D numpy array
np.array([1,2,3,np.nan,5,6,7,np.nan])
62. How to compute the euclidean distance between two arrays?
Q. Compute the euclidean distance between two arrays a and b .
63. How to find all the local maxima (or peaks) in a 1d array?
Q. Find all the peaks in a 1D numpy array a . Peaks are points surrounded by smaller values on both sides.
a = np.array([1, 3, 7, 1, 2, 6, 0, 1])
where, 2 and 5 are the positions of peak values 7 and 6.
64. How to subtract a 1d array from a 2d array, where each item of 1d array subtracts from respective row?
Q. Subtract the 1d array b_1d from the 2d array a_2d , such that each item of b_1d subtracts from respective row of a_2d .
65. How to find the index of n'th repetition of an item in an array
Difficulty Level L2
Q. Find the index of 5th repetition of number 1 in x .
66. How to convert numpy's datetime64 object to datetime's datetime object?
Q. Convert numpy's datetime64 object to datetime's datetime object
67. How to compute the moving average of a numpy array?
Q. Compute the moving average of window size 3, for the given 1D array.
68. How to create a numpy array sequence given only the starting point, length and the step?
Q. Create a numpy array of length 10, starting from 5 and has a step of 3 between consecutive numbers
69. How to fill in missing dates in an irregular series of numpy dates?
Q. Given an array of a non-continuous sequence of dates. Make it a continuous sequence of dates, by filling in the missing dates.
70. How to create strides from a given 1D array?
Q. From the given 1d array arr , generate a 2d matrix using strides, with a window length of 4 and strides of 2, like [[0,1,2,3], [2,3,4,5], [4,5,6,7]..]
More Articles
How to convert python code to cython (and speed up 100x), how to convert python to cython inside jupyter notebooks, install opencv python – a comprehensive guide to installing “opencv-python”, install pip mac – how to install pip in macos: a comprehensive guide, scrapy vs. beautiful soup: which is better for web scraping, add python to path – how to add python to the path environment variable in windows, similar articles, complete introduction to linear regression in r, how to implement common statistical significance tests and find the p value, logistic regression – a complete tutorial with examples in r.
Subscribe to Machine Learning Plus for high value data science content
© Machinelearningplus. All rights reserved.

Machine Learning A-Z™: Hands-On Python & R In Data Science
Free sample videos:.

- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Python Exercise with Practice Questions and Solutions
- Python List Exercise
- Python String Exercise
- Python Tuple Exercise
- Python Dictionary Exercise
- Python Set Exercise
Python Matrix Exercises
- Python program to a Sort Matrix by index-value equality count
- Python Program to Reverse Every Kth row in a Matrix
- Python Program to Convert String Matrix Representation to Matrix
- Python - Count the frequency of matrix row length
- Python - Convert Integer Matrix to String Matrix
- Python Program to Convert Tuple Matrix to Tuple List
- Python - Group Elements in Matrix
- Python - Assigning Subsequent Rows to Matrix first row elements
- Adding and Subtracting Matrices in Python
- Python - Convert Matrix to dictionary
- Python - Convert Matrix to Custom Tuple Matrix
- Python - Matrix Row subset
- Python - Group similar elements into Matrix
- Python - Row-wise element Addition in Tuple Matrix
- Create an n x n square matrix, where all the sub-matrix have the sum of opposite corner elements as even
Python Functions Exercises
- Python splitfields() Method
- How to get list of parameters name from a function in Python?
- How to Print Multiple Arguments in Python?
- Python program to find the power of a number using recursion
- Sorting objects of user defined class in Python
- Assign Function to a Variable in Python
- Returning a function from a function - Python
- What are the allowed characters in Python function names?
- Defining a Python function at runtime
- Explicitly define datatype in a Python function
- Functions that accept variable length key value pair as arguments
- How to find the number of arguments in a Python function?
- How to check if a Python variable exists?
- Python - Get Function Signature
- Python program to convert any base to decimal by using int() method
Python Lambda Exercises
- Python - Lambda Function to Check if value is in a List
- Difference between Normal def defined function and Lambda
- Python: Iterating With Python Lambda
- How to use if, else & elif in Python Lambda Functions
- Python - Lambda function to find the smaller value between two elements
- Lambda with if but without else in Python
- Python Lambda with underscore as an argument
- Difference between List comprehension and Lambda in Python
- Nested Lambda Function in Python
- Python lambda
- Python | Sorting string using order defined by another string
- Python | Find fibonacci series upto n using lambda
- Overuse of lambda expressions in Python
- Python program to count Even and Odd numbers in a List
- Intersection of two arrays in Python ( Lambda expression and filter function )
Python Pattern printing Exercises
- Simple Diamond Pattern in Python
- Python - Print Heart Pattern
- Python program to display half diamond pattern of numbers with star border
- Python program to print Pascal's Triangle
- Python program to print the Inverted heart pattern
- Python Program to print hollow half diamond hash pattern
- Program to Print K using Alphabets
- Program to print half Diamond star pattern
- Program to print window pattern
- Python Program to print a number diamond of any given size N in Rangoli Style
- Python program to right rotate n-numbers by 1
- Python Program to print digit pattern
- Print with your own font using Python !!
- Python | Print an Inverted Star Pattern
- Program to print the diamond shape
Python DateTime Exercises
- Python - Iterating through a range of dates
- How to add time onto a DateTime object in Python
- How to add timestamp to excel file in Python
- Convert string to datetime in Python with timezone
- Isoformat to datetime - Python
- Python datetime to integer timestamp
- How to convert a Python datetime.datetime to excel serial date number
- How to create filename containing date or time in Python
- Convert "unknown format" strings to datetime objects in Python
- Extract time from datetime in Python
- Convert Python datetime to epoch
- Python program to convert unix timestamp string to readable date
- Python - Group dates in K ranges
- Python - Divide date range to N equal duration
- Python - Last business day of every month in year
Python OOPS Exercises
- Get index in the list of objects by attribute in Python
- Python program to build flashcard using class in Python
- How to count number of instances of a class in Python?
- Shuffle a deck of card with OOPS in Python
- What is a clean and Pythonic way to have multiple constructors in Python?
- How to Change a Dictionary Into a Class?
- How to create an empty class in Python?
- Student management system in Python
- How to create a list of object in Python class
Python Regex Exercises
- Validate an IP address using Python without using RegEx
- Python program to find the type of IP Address using Regex
- Converting a 10 digit phone number to US format using Regex in Python
- Python program to find Indices of Overlapping Substrings
- Python program to extract Strings between HTML Tags
- Python - Check if String Contain Only Defined Characters using Regex
- How to extract date from Excel file using Pandas?
- Python program to find files having a particular extension using RegEx
- How to check if a string starts with a substring using regex in Python?
- How to Remove repetitive characters from words of the given Pandas DataFrame using Regex?
- Extract punctuation from the specified column of Dataframe using Regex
- Extract IP address from file using Python
- Python program to Count Uppercase, Lowercase, special character and numeric values using Regex
- Categorize Password as Strong or Weak using Regex in Python
- Python - Substituting patterns in text using regex
Python LinkedList Exercises
- Python program to Search an Element in a Circular Linked List
- Implementation of XOR Linked List in Python
- Pretty print Linked List in Python
- Python Library for Linked List
- Python | Stack using Doubly Linked List
- Python | Queue using Doubly Linked List
- Program to reverse a linked list using Stack
- Python program to find middle of a linked list using one traversal
- Python Program to Reverse a linked list
Python Searching Exercises
- Binary Search (bisect) in Python
- Python Program for Linear Search
- Python Program for Anagram Substring Search (Or Search for all permutations)
- Python Program for Binary Search (Recursive and Iterative)
- Python Program for Rabin-Karp Algorithm for Pattern Searching
- Python Program for KMP Algorithm for Pattern Searching
Python Sorting Exercises
- Python Code for time Complexity plot of Heap Sort
- Python Program for Stooge Sort
- Python Program for Recursive Insertion Sort
- Python Program for Cycle Sort
- Bisect Algorithm Functions in Python
- Python Program for BogoSort or Permutation Sort
- Python Program for Odd-Even Sort / Brick Sort
- Python Program for Gnome Sort
- Python Program for Cocktail Sort
- Python Program for Bitonic Sort
- Python Program for Pigeonhole Sort
- Python Program for Comb Sort
- Python Program for Iterative Merge Sort
- Python Program for Binary Insertion Sort
- Python Program for ShellSort
Python DSA Exercises
- Saving a Networkx graph in GEXF format and visualize using Gephi
- Dumping queue into list or array in Python
- Python program to reverse a stack
- Python - Stack and StackSwitcher in GTK+ 3
- Multithreaded Priority Queue in Python
- Python Program to Reverse the Content of a File using Stack
- Priority Queue using Queue and Heapdict module in Python
- Box Blur Algorithm - With Python implementation
- Python program to reverse the content of a file and store it in another file
- Check whether the given string is Palindrome using Stack
- Take input from user and store in .txt file in Python
- Change case of all characters in a .txt file using Python
- Finding Duplicate Files with Python
Python File Handling Exercises
- Python Program to Count Words in Text File
- Python Program to Delete Specific Line from File
- Python Program to Replace Specific Line in File
- Python Program to Print Lines Containing Given String in File
- Python - Loop through files of certain extensions
- Compare two Files line by line in Python
- How to keep old content when Writing to Files in Python?
- How to get size of folder using Python?
- How to read multiple text files from folder in Python?
- Read a CSV into list of lists in Python
- Python - Write dictionary of list to CSV
- Convert nested JSON to CSV in Python
- How to add timestamp to CSV file in Python
Python CSV Exercises
- How to create multiple CSV files from existing CSV file using Pandas ?
- How to read all CSV files in a folder in Pandas?
- How to Sort CSV by multiple columns in Python ?
- Working with large CSV files in Python
- How to convert CSV File to PDF File using Python?
- Visualize data from CSV file in Python
- Python - Read CSV Columns Into List
- Sorting a CSV object by dates in Python
- Python program to extract a single value from JSON response
- Convert class object to JSON in Python
- Convert multiple JSON files to CSV Python
- Convert JSON data Into a Custom Python Object
- Convert CSV to JSON using Python
Python JSON Exercises
- Flattening JSON objects in Python
- Saving Text, JSON, and CSV to a File in Python
- Convert Text file to JSON in Python
- Convert JSON to CSV in Python
- Convert JSON to dictionary in Python
- Python Program to Get the File Name From the File Path
- How to get file creation and modification date or time in Python?
- Menu driven Python program to execute Linux commands
- Menu Driven Python program for opening the required software Application
- Open computer drives like C, D or E using Python
Python OS Module Exercises
- Rename a folder of images using Tkinter
- Kill a Process by name using Python
- Finding the largest file in a directory using Python
- Python - Get list of running processes
- Python - Get file id of windows file
- Python - Get number of characters, words, spaces and lines in a file
- Change current working directory with Python
- How to move Files and Directories in Python
- How to get a new API response in a Tkinter textbox?
- Build GUI Application for Guess Indian State using Tkinter Python
- How to stop copy, paste, and backspace in text widget in tkinter?
- How to temporarily remove a Tkinter widget without using just .place?
- How to open a website in a Tkinter window?
Python Tkinter Exercises
- Create Address Book in Python - Using Tkinter
- Changing the colour of Tkinter Menu Bar
- How to check which Button was clicked in Tkinter ?
- How to add a border color to a button in Tkinter?
- How to Change Tkinter LableFrame Border Color?
- Looping through buttons in Tkinter
- Visualizing Quick Sort using Tkinter in Python
- How to Add padding to a tkinter widget only on one side ?
- Python NumPy - Practice Exercises, Questions, and Solutions
- Pandas Exercises and Programs
- How to get the Daily News using Python
- How to Build Web scraping bot in Python
- Scrape LinkedIn Using Selenium And Beautiful Soup in Python
- Scraping Reddit with Python and BeautifulSoup
- Scraping Indeed Job Data Using Python
Python Web Scraping Exercises
- How to Scrape all PDF files in a Website?
- How to Scrape Multiple Pages of a Website Using Python?
- Quote Guessing Game using Web Scraping in Python
- How to extract youtube data in Python?
- How to Download All Images from a Web Page in Python?
- Test the given page is found or not on the server Using Python
- How to Extract Wikipedia Data in Python?
- How to extract paragraph from a website and save it as a text file?
- Automate Youtube with Python
- Controlling the Web Browser with Python
- How to Build a Simple Auto-Login Bot with Python
- Download Google Image Using Python and Selenium
- How To Automate Google Chrome Using Foxtrot and Python
Python Selenium Exercises
- How to scroll down followers popup in Instagram ?
- How to switch to new window in Selenium for Python?
- Python Selenium - Find element by text
- How to scrape multiple pages using Selenium in Python?
- Python Selenium - Find Button by text
- Web Scraping Tables with Selenium and Python
- Selenium - Search for text on page
- Python Projects - Beginner to Advanced
Python NumPy – Practice Exercises, Questions, and Solutions
Python NumPy is a general-purpose array processing package. It provides fast and versatile n-dimensional arrays and tools for working with these arrays. It provides various computing tools such as comprehensive mathematical functions, random number generator and it’s easy to use syntax makes it highly accessible and productive for programmers from any background.
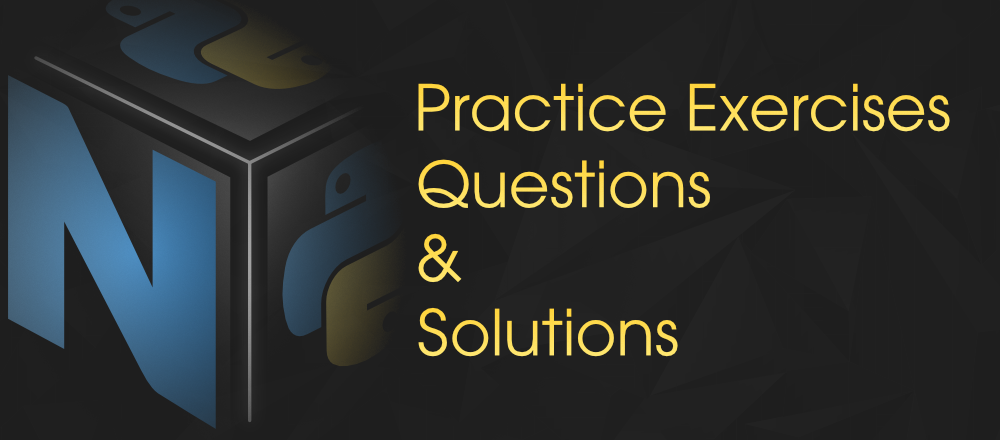
This NumPy exercise will help the learners to get a better understanding of NumPy arrays. This practice page consists of a huge set of NumPy programs like NumPy array, Matrix, handling indexing in NumPy, working with Mathematics. Statistics and all sort of frequently encountered problems.
Questions on NumPy Array
- How to create an empty and a full NumPy array?
- Create a Numpy array filled with all zeros
- Create a Numpy array filled with all ones
- Check whether a Numpy array contains a specified row
- How to Remove rows in Numpy array that contains non-numeric values?
- Remove single-dimensional entries from the shape of an array
- Find the number of occurrences of a sequence in a NumPy array
- Find the most frequent value in a NumPy array
- Combining a one and a two-dimensional NumPy Array
- How to build an array of all combinations of two NumPy arrays?
- How to add a border around a NumPy array?
- How to compare two NumPy arrays?
- How to check whether specified values are present in NumPy array?
- How to get all 2D diagonals of a 3D NumPy array?
- Flatten a Matrix in Python using NumPy
- Flatten a 2d numpy array into 1d array
- Move axes of an array to new positions
- Interchange two axes of an array
- NumPy – Fibonacci Series using Binet Formula
- Counts the number of non-zero values in the array
- Count the number of elements along a given axis
- Trim the leading and/or trailing zeros from a 1-D array
- Change data type of given numpy array
- Reverse a numpy array
- How to make a NumPy array read-only?
Questions on NumPy Matrix
- Get the maximum value from given matrix
- Get the minimum value from given matrix
- Find the number of rows and columns of a given matrix using NumPy
- Select the elements from a given matrix
- Find the sum of values in a matrix
- Calculate the sum of the diagonal elements of a NumPy array
- Ways to add row/columns in numpy array
- Matrix Multiplication in NumPy
- Get the eigen values of a matrix
- How to Calculate the determinant of a matrix using NumPy?
- How to inverse a matrix using NumPy
- How to count the frequency of unique values in NumPy array?
- Multiply matrices of complex numbers using NumPy in Python
- Compute the outer product of two given vectors using NumPy in Python
- Calculate inner, outer, and cross products of matrices and vectors using NumPy
- Compute the covariance matrix of two given NumPy arrays
- Convert covariance matrix to correlation matrix using Python
- Compute the Kronecker product of two mulitdimension NumPy arrays
- Convert the matrix into a list
Questions on NumPy Indexing
- Replace NumPy array elements that doesn’t satisfy the given condition
- Return the indices of elements where the given condition is satisfied
- Replace NaN values with average of columns
- Replace negative value with zero in numpy array
- How to get values of an NumPy array at certain index positions?
- Find indices of elements equal to zero in a NumPy array
- How to Remove columns in Numpy array that contains non-numeric values?
- How to access different rows of a multidimensional NumPy array?
- Get row numbers of NumPy array having element larger than X
- Get filled the diagonals of NumPy array
- Check elements present in the NumPy array
- Combined array index by index
Questions on NumPy Linear Algebra
- Find a matrix or vector norm using NumPy
- Calculate the QR decomposition of a given matrix using NumPy
- Compute the condition number of a given matrix using NumPy
- Compute the eigenvalues and right eigenvectors of a given square array using NumPy?
- Calculate the Euclidean distance using NumPy
Questions on NumPy Random
- Create a Numpy array with random values
- How to choose elements from the list with different probability using NumPy?
- How to get weighted random choice in Python?
- Generate Random Numbers From The Uniform Distribution using NumPy
- Get Random Elements form geometric distribution
- Get Random elements from Laplace distribution
- Return a Matrix of random values from a uniform distribution
- Return a Matrix of random values from a Gaussian distribution
Questions on NumPy Sorting and Searching
- How to get the indices of the sorted array using NumPy in Python?
- Finding the k smallest values of a NumPy array
- How to get the n-largest values of an array using NumPy?
- Sort the values in a matrix
- Filter out integers from float numpy array
- Find the indices into a sorted array
Questions on NumPy Mathematics
- How to get element-wise true division of an array using Numpy?
- How to calculate the element-wise absolute value of NumPy array?
- Compute the negative of the NumPy array
- Multiply 2d numpy array corresponding to 1d array
- Computes the inner product of two arrays
- Compute the nth percentile of the NumPy array
- Calculate the n-th order discrete difference along the given axis
- Calculate the sum of all columns in a 2D NumPy array
- Calculate average values of two given NumPy arrays
- How to compute numerical negative value for all elements in a given NumPy array?
- How to get the floor, ceiling and truncated values of the elements of a numpy array?
- How to round elements of the NumPy array to the nearest integer?
- Find the round off the values of the given matrix
- Determine the positive square-root of an array
- Evaluate Einstein’s summation convention of two multidimensional NumPy arrays
Questions on NumPy Statistics
- Compute the median of the flattened NumPy array
- Find Mean of a List of Numpy Array
- Calculate the mean of array ignoring the NaN value
- Get the mean value from given matrix
- Compute the variance of the NumPy array
- Compute the standard deviation of the NumPy array
- Compute pearson product-moment correlation coefficients of two given NumPy arrays
- Calculate the mean across dimension in a 2D NumPy array
- Calculate the average, variance and standard deviation in Python using NumPy
- Describe a NumPy Array in Python
Questions on Polynomial
- Define a polynomial function
- How to add one polynomial to another using NumPy in Python?
- How to subtract one polynomial to another using NumPy in Python?
- How to multiply a polynomial to another using NumPy in Python?
- How to divide a polynomial to another using NumPy in Python?
- Find the roots of the polynomials using NumPy
- Evaluate a 2-D polynomial series on the Cartesian product
- Evaluate a 3-D polynomial series on the Cartesian product
Questions on NumPy Strings
- Repeat all the elements of a NumPy array of strings
- How to split the element of a given NumPy array with spaces?
- How to insert a space between characters of all the elements of a given NumPy array?
- Find the length of each string element in the Numpy array
- Swap the case of an array of string
- Change the case to uppercase of elements of an array
- Change the case to lowercase of elements of an array
- Join String by a separator
- Check if two same shaped string arrayss one by one
- Count the number of substrings in an array
- Find the lowest index of the substring in an array
- Get the boolean array when values end with a particular character
More Questions on NumPy
- Different ways to convert a Python dictionary to a NumPy array
- How to convert a list and tuple into NumPy arrays?
- Ways to convert array of strings to array of floats
- Convert a NumPy array into a csv file
- How to Convert an image to NumPy array and save it to CSV file using Python?
- How to save a NumPy array to a text file?
- Load data from a text file
- Plot line graph from NumPy array
- Create Histogram using NumPy
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
Fundamentals of Machine Learning and Engineering
Exploring algorithms and concepts, tutorial - multivariate linear regression with numpy.
Welcome to one more tutorial! In the last post (see here ) we saw how to do a linear regression on Python using barely no library but native functions (except for visualization).
In this exercise, we will see how to implement a linear regression with multiple inputs using Numpy. We will also use the Gradient Descent algorithm to train our model.
The first step is to import all the necessary libraries. The ones we will use are:
- Numpy - for numerical calculations;
- Pandas - to read csv and data processing;
- Matplotlib - for visualization
Now import the data that we want to work on. This data (hypothetical) consists in the following information from real state properties:
- Size (the size of the housing in square meters);
- Nr Bedrooms -> the number of bedrooms;
- Nr Bathrooms -> the number of bathrooms
- Price -> The price of the house, in terms of thousands of dollars (or any other currency since the data is hypothetical)
Hypothesis The price is linearly correlated with the size, nr of bedrooms and nr of bathrooms of a housing.
We will check validity of the above hypothesis through linear regression.
Pandas function read_csv() is used to read the csv file ‘housingprices.csv’ and place it as a dataframe.
A good practice, before performing any computation, is to check wheter the data contains invalued values (such as NaNs - not a number). This can be done using pd.isna() function, which returns a dataframe of True or False values. Since we want to summarize the results for each column initially and know wheter there is AT LEAST one invalid value, we can use the any() function, which returns True if there is any invalid number, otherwise False.
No invalid value was found in the dataframe.
Split the dataset into inputs (x) and output(y). Use the method values to transform from a DataFrame object to an array object, which can efficiently managed by Numpy library.
Let’s generate a simple visualization the price in relation to each input variable.
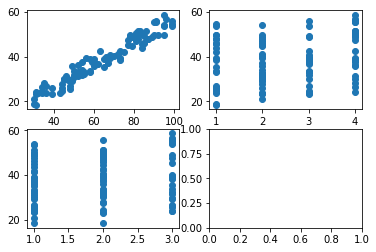
Linear correlation can be evaluated through Pearson’s coefficient, which returns a value between 0 and 1. 0 means there is no correlation, while 1 means perfect correlation. Everything in betweeen indicates that the data is somehow correlated, though usually a correlation of more than 0.8 is expected for a variable to be considered a predictor, i.e an input to a Machine Learning model.
Results above shows that only the size shows high correlation with the price. Even though, we will keep the other variables as predictor, for the sake of this exercise of a multivariate linear regression.
Add a bias column to the input vector. This is a column of ones so when we calibrate the parameters it will also multiply such bias.
Another important pre-processing is data normalization. In multivariate regression, the difference in the scale of each variable may cause difficulties for the optimization algorithm to converge, i.e to find the best optimum according the model structure. This procedure is also known as Feature Scaling .
Now we are ready to start working on the model. It is reasonable to give an initial guess on the parameters and check this initial guess makes sense or is it complete nonsense. In this exercise, the choosen vector of parameters $\theta$ is
Notice that there are 4 parameters, which corresponds to the bias + the input variables from the data. The linear regression model works according the following formula.
Thus, $X$ is the input matrix with dimension (99,4), while the vector $theta$ is a vector of $(4,1)$, thus the resultant matrix has dimension $(99,1)$, which indicates that our calculation process is correct.
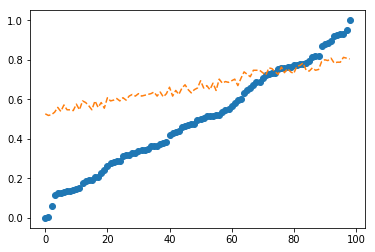
Create a function grad() to compute the necessary gradients of the cost function. This is explained with higher details in the other post I mentioned in the beginning of this one. But only to remember, the gradient can be calculated as:
Where $j=0,1,..,3$ since there are four predictors. This will produce a derivative for each input $j$, thus the complete gradient consists on the vector:
Similarly, calculate the cost function, also known as objective function, which can be expressed as the sum of the squared errors, as follows.
We are ready to implement the Gradient Descent algorithm! The steps of this algorithm consists of:
- Obtain the gradients of the cost function according the actual value of the parameters;
- Calculate the cost to keep track of it;
- Update the parameters according the following schedule:
Where the superscript $i$ refers to the current iteration. Then the iterative steps are repeated until the algorithm converges.
In the above code, a maximum number of iterations is fixed. This avoid the algorithm to loop infinitely. Additionally, the iteration process is stopped if the following criteria is met at any point.
Where TOL is a tolerance, i.e a maximum difference between the values of the parameters between iterations so it can be stated that the values converged.
Next, evaluate the Gradient Descent to determine the optimum set of parameters for the linear regression.
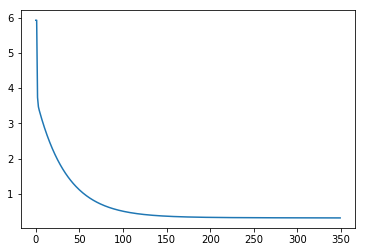
Observe in the plot above how the cost function J drastically reduces at the initial iterations, converging to a value much smaller than the initial one. By using an appropriate Tolerance TOL, the iteration process was halted at less than 350 iterations, though the maximum number was initially fixed to 1000.
We can perform predictions on the training set using the following code.
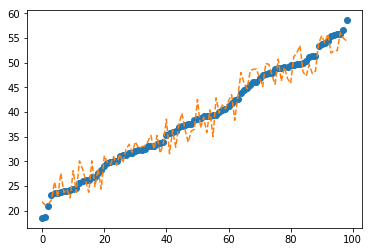
The following function is to used to get an input, normalize it, perform predictions using the values of $\theta$ encountered, and denormalizing the output to come back to the original range.
Let’s use our model to predict the price of a house with 73 square meters, 1 bedroom and 1 bathroom.
To confirm that the model is statistically significant, use the Pearson correlation and evaluate the predicted output against the observed one.
Notice here that the value of pearson correlation is 0.97 which indicates high correlation. The second value (1.93e-65) is the p-value. It must be a very low value for us to reject the null hypothesis, i,e there is no initial hypothesis of linear correlation. Assuming 1% significance level, we have: 0.01 >> 1.93e-65 thus we can reject the null hypothesis thus indicating linear correlation between the prediction and observations.
The Jupyter notebook for this tutorial can be downloaded from here ! If you want it as python code, download it here ! See you next post!
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python variables - assign multiple values, many values to multiple variables.
Python allows you to assign values to multiple variables in one line:
Note: Make sure the number of variables matches the number of values, or else you will get an error.
One Value to Multiple Variables
And you can assign the same value to multiple variables in one line:
Unpack a Collection
If you have a collection of values in a list, tuple etc. Python allows you to extract the values into variables. This is called unpacking .
Unpack a list:
Learn more about unpacking in our Unpack Tuples Chapter.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
numpy.multiply #
Multiply arguments element-wise.
Input arrays to be multiplied. If x1.shape != x2.shape , they must be broadcastable to a common shape (which becomes the shape of the output).
A location into which the result is stored. If provided, it must have a shape that the inputs broadcast to. If not provided or None, a freshly-allocated array is returned. A tuple (possible only as a keyword argument) must have length equal to the number of outputs.
This condition is broadcast over the input. At locations where the condition is True, the out array will be set to the ufunc result. Elsewhere, the out array will retain its original value. Note that if an uninitialized out array is created via the default out=None , locations within it where the condition is False will remain uninitialized.
For other keyword-only arguments, see the ufunc docs .
The product of x1 and x2 , element-wise. This is a scalar if both x1 and x2 are scalars.
Equivalent to x1 * x2 in terms of array broadcasting.
The * operator can be used as a shorthand for np.multiply on ndarrays.

COMMENTS
We can select (and return) a specific element from a NumPy array in the same way that we could using a normal Python list: using square brackets. An example is below: arr[0] #Returns 0.69. We can also reference multiple elements of a NumPy array using the colon operator. For example, the index [2:] selects every element from index 2 onwards.
ndarrays. #. ndarrays can be indexed using the standard Python x[obj] syntax, where x is the array and obj the selection. There are different kinds of indexing available depending on obj : basic indexing, advanced indexing and field access. Most of the following examples show the use of indexing when referencing data in an array.
Accessing Multiple Fields# One can index and assign to a structured array with a multi-field index, where the index is a list of field names. Warning. ... Normally in numpy >= 1.14, assignment of one structured array to another copies fields "by position", meaning that the first field from the src is copied to the first field of the dst ...
3. Consider the multiple assignment x[0],y = y,x[0]. Applied to each of the four below cases, this gives four different results. It appears that the multiple assignment of lists is smarter (going through a temporary variable automatically) than the multiple assignment of Numpy arrays.
You can also swap the values of multiple variables in the same way. See the following article for details: Swap values in a list or values of variables in Python; Assign the same value to multiple variables. You can assign the same value to multiple variables by using = consecutively.
numpy.put. #. Replaces specified elements of an array with given values. The indexing works on the flattened target array. put is roughly equivalent to: Target array. Target indices, interpreted as integers. Values to place in a at target indices. If v is shorter than ind it will be repeated as necessary. Specifies how out-of-bounds indices ...
How to Create Multi-Dimensional Arrays Using NumPy. To create a multi-dimensional array using NumPy, we can use the np.array() function and pass in a nested list of values as an argument. The outer list represents the rows of the array, and the inner lists represent the columns. Here is an example of how to create a 2-dimensional array using NumPy:
An instructive first step is to visualize, given the patch size and image shape, what a higher-dimensional array of patches would look like. We have a 2d array img with shape (254, 319) and a (10, 10) 2d patch. This means our output shape (before taking the mean of each "inner" 10x10 array) would be: Python.
101 Practice exercises with pandas. 1. Import numpy as np and see the version. Difficulty Level: L1. Q. Import numpy as np and print the version number. 2. How to create a 1D array? Difficulty Level: L1. Q. Create a 1D array of numbers from 0 to 9.
The elements of both a and a.T get traversed in the same order, namely the order they are stored in memory, whereas the elements of a.T.copy(order='C') get visited in a different order because they have been put into a different memory layout.. Controlling iteration order#. There are times when it is important to visit the elements of an array in a specific order, irrespective of the ...
You can even assign multiple values to an equal number of variables in a single line, commonly known as parallel assignment. Additionally, you can simultaneously assign the values in an iterable to a comma-separated group of variables in what's known as an iterable unpacking operation. ... However, the numpy and pandas names are not.
Python NumPy is a general-purpose array processing package. It provides fast and versatile n-dimensional arrays and tools for working with these arrays. It provides various computing tools such as comprehensive mathematical functions, random number generator and it's easy to use syntax makes it highly accessible and productive for programmers from any background.
delete (arr, obj [, axis]) Return a new array with sub-arrays along an axis deleted. insert (arr, obj, values [, axis]) Insert values along the given axis before the given indices. append (arr, values [, axis]) Append values to the end of an array. resize (a, new_shape) Return a new array with the specified shape.
In this exercise, we will see how to implement a linear regression with multiple inputs using Numpy. We will also use the Gradient Descent algorithm to train our model. The first step is to import all the necessary libraries. The ones we will use are: Numpy - for numerical calculations; Pandas - to read csv and data processing;
Notice when you perform operations with two arrays of the same dtype: uint32, the resulting array is the same type.When you perform operations with different dtype, NumPy will assign a new type that satisfies all of the array elements involved in the computation, here uint32 and int32 can both be represented in as int64.. The default NumPy behavior is to create arrays in either 32 or 64-bit ...
Learn NumPy Tutorial Learn Pandas Tutorial Learn SciPy Tutorial Learn Matplotlib Tutorial Learn ... Python allows you to assign values to multiple variables in one line: Example. x, y, z = "Orange", "Banana", "Cherry" print(x)
numpy: convert multiple assignments to a single one using OR. 1. Assigning Numpy array to variables. 0. Can I get a similar behavior with numpy broadcasting to the following array assignment in matlab. Hot Network Questions Integral's computation does not match WolframAlpha result
As you can see, my array contains floats, positive, negative, zeros and NaNs. I would like to re-assign (re-class) the values in the array based on multiple if statements. I've read many answers and docs but all of which I've seen refer to a simple one or two conditions which can be easily be resolved using np.where for example. I have multiple ...
numpy.multiply. #. Multiply arguments element-wise. Input arrays to be multiplied. If x1.shape != x2.shape, they must be broadcastable to a common shape (which becomes the shape of the output). A location into which the result is stored. If provided, it must have a shape that the inputs broadcast to. If not provided or None, a freshly-allocated ...