(React) Expected an assignment or function call and instead saw an expression
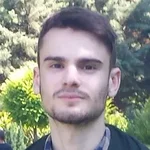
Last updated: Apr 6, 2024 Reading time · 3 min
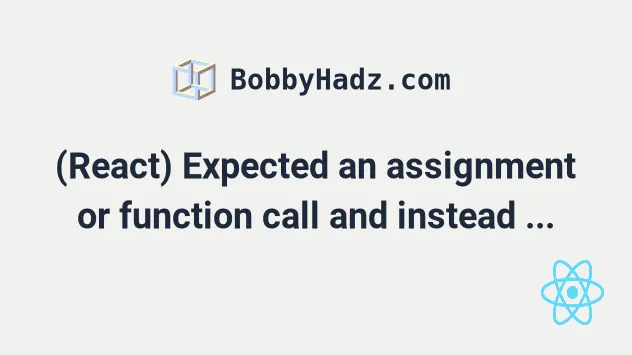

# (React) Expected an assignment or function call and instead saw an expression
The React.js error "Expected an assignment or function call and instead saw an expression" occurs when we forget to return a value from a function.
To solve the error, make sure to explicitly use a return statement or implicitly return using an arrow function.
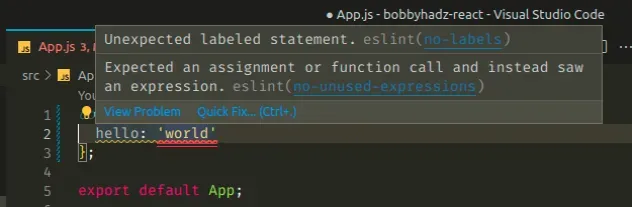
Here are 2 examples of how the error occurs.
In the App component, the error is caused in the Array.map() method.
The issue is that we aren't returning anything from the callback function we passed to the map() method.
The issue in the mapStateToProps function is the same - we forgot to return a value from the function.
# Solve the error using an explicit return
To solve the error, we have to either use an explicit return statement or implicitly return a value using an arrow function.
Here is an example of how to solve the error using an explicit return .
We solved the issue in our map() method by explicitly returning. This is necessary because the Array.map() method returns an array containing all of the values that were returned from the callback function we passed to it.
# Solve the error using an implicit return
An alternative approach is to use an implicit return with an arrow function.
We used an implicit arrow function return for the App component.
If we are using an implicit return to return an object, we have to wrap the object in parentheses.
An easy way to think about it is - when you use curly braces without wrapping them in parentheses, you are declaring a block of code (like in an if statement).
When used without parentheses, you have a block of code, not an object.
If you believe that the Eslint rule shouldn't be showing an error, you can turn it off for a single line, by using a comment.
The comment should be placed right above the line where the error is caused.
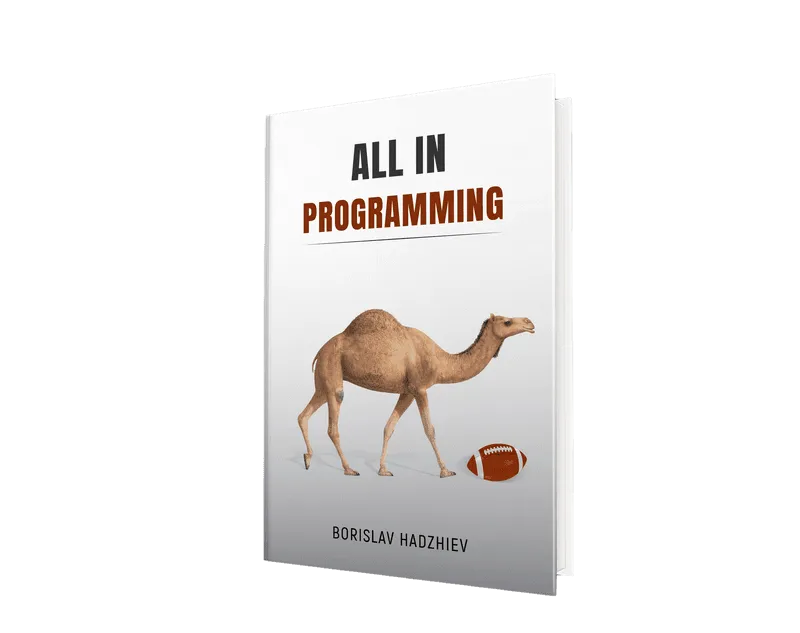
Borislav Hadzhiev
Web Developer
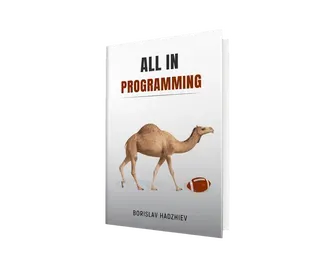
Copyright © 2024 Borislav Hadzhiev
How to Use the Ternary Operator in JavaScript – Explained with Examples
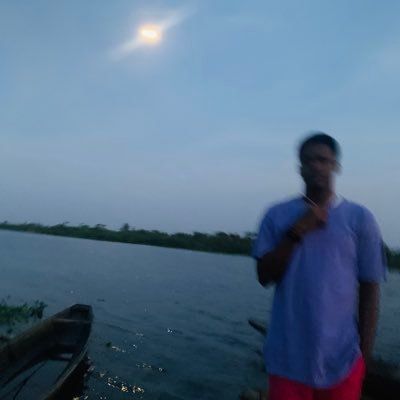
Tired of bulky if-else statements? JavaScript's ternary operator offers a powerful solution. This handy tool lets you condense complex conditional logic into a single line, making your code cleaner, more elegant, and efficient.
In this article, we'll take a deep dive into the ternary operator, understanding its syntax and showcasing real-world examples to help you understand how it works to harness its full potential.
Here is What We'll Cover:
What is a ternary operator, how to use the ternary operator.
- How to Refactor if-else Statements to Ternary operator
How to Chain Ternary Operators
- Best Practices when using the Ternary Operator
A ternary operator is a conditional operator in JavaScript that evaluates a conditional expression and returns either a truthy or falsy value.
To understand how this works, let's take a closer look at its syntax below:
From the syntax above, the condionalExpression is the expression that serves as the evaluation point, determining either a truthy or falsy value.
Following the ? (question mark), the value provided is returned in case the expression evaluates to truthy, whereas the value following the : (colon) is returned if the expression results in a falsy outcome.
The truthyValue and falsyValue can be anything in JavaScript. It can encompass various entities such as functions, values stored in variables, objects, numbers, strings, and more. The ternary operator grants you the flexibility to return any desired value, offering versatility in your code.
Now that we've examined the syntax and its functionality, let's explore how to use the ternary operator to deepen our understanding.
Consider this scenario: we're building a gaming platform that only allows users that are aged 18 and above. We'll design a function to check a user's age. If they're under 18, they'll be denied access; otherwise, they'll gain entry to the platform.
From the code snippet above, we created a function, canAccessPlatform , which evaluates whether a user, represented by their age parameter, meets the requirement to access the platform.
It utilizes a ternary operator to determine if the age is 18 or older, assigning true to shouldAccess if the condition is met, and false otherwise. Finally, it returns the value of shouldAccess , indicating whether the user can access the platform or not.
If the age is 18 or older, the expression becomes true, so the operator returns true after the ? . Otherwise, it returns false. This result is saved in a variable and then returned from the function.
While this basic use case simplifies code and improves readability by replacing unnecessary if-else blocks, it's important to use it sparingly to avoid cluttering and complicating your code. Later, we'll discuss best practices for using the ternary operator.
Here's another example illustrating the use of the ternary operator. We'll create a function to determine whether a number is even or odd. Check out the code snippet below:
From the code snippet above:
- We define a function checkEvenOrOdd that takes a number parameter.
- Inside the function, we use the ternary operator to check if the number is even or odd.
- If the number modulo 2 equals 0 (meaning it's divisible by 2 with no remainder), then the condition evaluates to true, and the string "even" is assigned to the result variable.
- If the condition evaluates to false (meaning the number is odd), the string "odd" is assigned to result .
- Finally, the function returns the value of result , which indicates whether the number is even or odd.
This code shows how the ternary operator quickly checks if a number is even or odd, making the code easier to read and understand.
How to Refactor if-else Statements to Ternary Operator
An advantage of the ternary operator is avoiding unnecessary if-else blocks, which can complicate code readability and maintenance. In this section, we'll refactor some if-else statements into ternary operations, providing a clearer understanding of how to use ternary operators effectively.
Let's start with our first example:
This function, decideActivity , takes a weather parameter and determines the appropriate activity based on the weather condition.
If the weather is "sunny", it suggests to "go out". Otherwise, it advises to "stay in". When we call the function with different weather conditions like "raining" or "snowing", it outputs the corresponding activity recommendation using console.log() .
For instance, calling decideActivity("raining") will output "stay in". Similarly, decideActivity("snowing") also outputs "stay in". When decideActivity("sunny") is called, it outputs "go out". This straightforward function helps decide on activities based on the weather condition provided.
Now, we can refactor these blocks of code to make them look simpler and neater. Let's see how to do that below:
From the code sample above, this function, decideActivity , uses the ternary operator to quickly determine the activity based on the weather condition. It checks if the weather is "sunny" and assigns "go out" if true, otherwise "stay in".
We've simplified the if-else statements into a one-liner ternary operator. This makes our code cleaner, clearer, and easier to read.
Let take a look at another example:
Let's explain what the code above is doing:
- Function Definition : We begin by defining a function named checkNumber that takes a single parameter called number .
- Variable Declaration : Inside the function, we declare a variable named result without assigning any value to it yet. This variable will store the result of our check.
- Conditional Statement (if-else) : We have a conditional statement that checks whether the number parameter is greater than 0.
- If the condition is true (meaning the number is positive), we assign the string "positive" to the result variable.
- If the condition is false (meaning the number is not positive, (meaning it is either negative or zero), we assign the string "non-positive" to the result variable.
- Return Statement : Finally, we return the value stored in the result variable.
- Function Calls :We then call the checkNumber function twice with different arguments: 5 and -2.
When we call checkNumber(5) , the function returns "positive", which is then logged to the console.
Similarly, when we call checkNumber(-2) , the function returns "non-positive", which is again logged to the console.
This function efficiently determines whether a number is positive or non-positive and provides the appropriate result based on the condition.
Let's simplify and improve the code by rewriting it using a ternary operator.
Great job! By refactoring the function and utilizing the ternary operator for conditional evaluation, we've achieved cleaner, more concise, and readable code.
This code, using the ternary operator, feels more concise and elegant. It efficiently determines if a number is positive or non-positive, making the code cleaner and easier to understand. When we call checkNumber(5) , it returns "positive", while checkNumber(-2) returns "non-positive". Overall, the ternary operator enhances the code's readability.
When dealing with conditional checks, sometimes a single condition isn't enough. In such cases, we use 'else-if' statements alongside 'if/else' to incorporate multiple conditions.
Let's take a look at the syntax:
This can be translated into an if/else chain:
Let's explore an example below:
This code above defines a function called checkNumber that takes a number parameter and determines its status (positive, zero, or negative). It utilizes an if-else block with one else-if statement to evaluate the number's value. If the number is greater than 0, it's considered positive and if it's equal to 0, it's zero. Otherwise, it's negative. The function returns the result.
Let's refactor this code using a ternary operator to achieve the same functionality.
That's it! We've refactored the function, and upon closer examination, we can observe that the operators are chained together. Now, let's explore how the chained ternary operator works in the checkNumber function.
In the first ternary operator:
- The first part number > 0 checks if the number is greater than 0.
- If it's true, the expression returns "Positive".
In the second ternary operator (chained):
- If the first condition is false (meaning the number is not greater than 0), it moves to the next part of the expression: number === 0 .
- This part checks if the number is equal to 0.
- If it's true, the expression returns "Zero".
And the default value:
- If neither of the above conditions is true (meaning the number is not greater than 0 and not equal to 0), it defaults to the last part of the expression: "Negative" .
- This part acts as the default value if none of the preceding conditions are met.
In summary, the chained ternary operator evaluates multiple conditions in a single line of code. It checks each condition sequentially, and the first condition that evaluates to true determines the result of the entire expression. This allows for concise and efficient conditional logic.
Let's examine another example of a chained ternary operator.
In the given code sample, the ternary operators are chained together to provide different drink suggestions based on the age provided. Each conditional expression in the chain evaluates a specific age range.
If the first condition is true (truthy), it returns 'Enjoy a cocktail'. If false (falsy), it moves to the next conditional expression, and so on. This chaining process continues until a condition evaluates to true. If none of the conditions in the chain are true, the last value is returned as a fallback, similar to the 'else' block in an if/else statement.
The concept of 'chaining' ternary operators involves linking conditional expressions based on the value of the previous expression. This can be compared to the else if structure in an if/else statement, providing a concise way to handle multiple conditions in JavaScript.
Best Practices when Using the Ternary Operator
Using the ternary operator efficiently can significantly enhance code readability and conciseness. In this section, we'll explore key best practices for utilizing the ternary operator effectively.
- Keep it simple and readable : Write concise expressions that are easy to understand at a glance. Avoid nesting too many ternary operators or writing overly complex conditions.
- Use for simple assignments: Ternary operators are ideal for simple assignments where there are only two possible outcomes based on a condition. For more complex scenarios, consider using if/else statements.
- Know when to use it : Use the ternary operator when you need to perform a simple conditional check and assign a value based on the result. It's particularly useful for assigning default values or determining the value of a variable based on a condition.
- Test thoroughly : Test your code thoroughly to ensure that the ternary operator behaves as expected under different conditions. Check for edge cases and validate the correctness of the assigned values.
- Avoid nested ternaries: While chaining ternaries is possible, excessive nesting can lead to code that is difficult to read. Prefer clarity and consider using if/else for complex conditions.
- Keep ternaries short: Aim to keep ternary expressions short and concise. Long ternaries can be difficult to read and understand, leading to code maintenance challenges.
These best practices outline guidelines for effectively utilizing the ternary operator. While they are not strict rules, they offer valuable insights to enhance the clarity and readability of your code.
As we conclude this article, you've gained a comprehensive understanding of the ternary operator—its application in daily coding tasks, converting if/else statements, chaining operators, and best practices. I'm confident that you've acquired valuable insights that will enhance your coding practices using the ternary operator.
Thank you for reading, and see you next time!
Contact information
Would you like to get in touch with me? Don't hesitate to reach out through any of the following channels:
- Twitter / X: @developeraspire
- Email: [email protected]
A Frontend Engineer with over 3 years of experience in the web development industry, I am passionate about creating engaging, user-friendly, and high-performing websites and web applications.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Ternary operator not working in React Challenge
In one of the React challenges an if/else statement was required in shouldComponentUpdate :
I thought I’d get clever and replace the if/else with a ternary operator:
and got the following error message:
Expected an assignment or function call and instead saw an expression
Could any one point out where I’ve gone wrong?

The ternary is just sitting there. You forgot to return it.
You could even drop the ternary and just return the condition.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Conditional (ternary) operator
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark ( ? ), then an expression to execute if the condition is truthy followed by a colon ( : ), and finally the expression to execute if the condition is falsy . This operator is frequently used as an alternative to an if...else statement.
An expression whose value is used as a condition.
An expression which is executed if the condition evaluates to a truthy value (one which equals or can be converted to true ).
An expression which is executed if the condition is falsy (that is, has a value which can be converted to false ).
Description
Besides false , possible falsy expressions are: null , NaN , 0 , the empty string ( "" ), and undefined . If condition is any of these, the result of the conditional expression will be the result of executing the expression exprIfFalse .
A simple example
Handling null values.
One common usage is to handle a value that may be null :
Conditional chains
The ternary operator is right-associative, which means it can be "chained" in the following way, similar to an if … else if … else if … else chain:
This is equivalent to the following if...else chain.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Nullish coalescing operator ( ?? )
- Optional chaining ( ?. )
- Making decisions in your code — conditionals
- Expressions and operators guide
Just Learn Code
Mastering the expected an assignment or function call error in reactjs.
React.js Error: “Expected an assignment or function call and instead saw an expression”
React.js is an open-source, front-end JavaScript library utilized for building user interfaces and UI components. With its ability to create reusable UI components, React.js has become a favorite tool for developers.
However, like any language-based tool, React.js has its own sets of rules. One of the most common errors encountered when working with React.js is the “Expected an assignment or function call and instead saw an expression” error.
In this article, we will explore this issue, its causes, and possible solutions.
The Issue with Forgetting to Return a Value from a Function
One of the most significant causes of this error is forgetting to return a value from a function. When using React.js, it’s essential to ensure that every function returns a value or a statement that will eventually lead to a return statement.
This error usually occurs when a function doesn’t return a value explicitly or doesn’t have an implicit return statement.
Examples in App Component and mapStateToProps Function
To understand better, let’s look at two examples: one in the App component and another in the mapStateToProps function. In the first example, we have the App component, which doesn’t return anything.
const App = () => {
Hi, welcome to my App!
The code above returns a value. However, a common mistake is to forget to include the return statement, which would lead to the “Expected an assignment or function call and instead saw an expression” error.
In the second example, we have the mapStateToProps function, which doesn’t return a value explicitly. const mapStateToProps = state => {
data: state.data
The mapStateToProps function is used with the react-redux library to connect the state from a React component to a Redux store.
However, if the function doesn’t return a value explicitly, the error will occur.
Solutions Include Using Explicit Return Statement or Implicit Return with Arrow Function
Now that we’ve seen the examples, let’s look at possible solutions. The first and most straightforward solution is to use an explicit return statement.
An explicit return statement is when we explicitly write “return” before returning a value. Here’s what the solution would look like when using explicit return statement.
The other solution is to use an implicit return with an arrow function. An implicit return statement is when there is no “return” keyword, but the expression returns a value.
Here’s what the solution would look like when using an implicit return with arrow function. const mapStateToProps = state => ({
As we can see, both solutions result in an explicit return statement that will resolve the issue and prevent “Expected an assignment or function call and instead saw an expression” error.
Solving the Error Using an Explicit Return
Another approach to solving this error is by ensuring to have an explicit return statement when using the Array.map() method. The Array.map() method is used to transform every element in an array, and it takes a callback function as a parameter.
The Importance of Explicitly Returning from Array.map() Method
The callback function used with the Array.map() method must return a new element value. However, it’s essential to ensure that each callback function returns with an explicit return statement.
If it doesn’t, it could lead to the “Expected an assignment or function call and instead saw an expression” error.
Example of Using Explicit Return Statement to Resolve Error
Here’s an example of using the explicit return statement. const newArray = oldArray.map((element) => {
return element * 2;
In the example above, every element in the “oldArray” is multiplied by two and returned with an explicit return statement, ultimately resolving the error.
In conclusion, “Expected an assignment or function call and instead saw an expression” is a common error when working with React.js. It’s usually caused by forgetting to return a value from a function or not having an explicit return statement when using the Array.map() method.
To resolve this error, we can use explicit return statements or implicit return with an arrow function. By understanding this error and its solutions, developers can create efficient and reliable React.js applications.
Solving the Error Using an Implicit Return
In the previous article, we discussed the “Expected an assignment or function call and instead saw an expression” error when working with React.js and how to solve it using an explicit return statement. However, there’s also another approach to resolving this error, and that is by using an implicit return with an arrow function.
Alternative Approach of Using Implicit Return with Arrow Function
An arrow function is a shorthand way to write a function in JavaScript. It provides a more concise syntax for writing functions and simplifies the code.
One of the benefits of using arrow functions is that they provide an implicit return statement if there is only one statement in the function body. Let’s take a look at an example of using an implicit return with an arrow function.
const addNumbers = (a, b) => a + b;
In the example above, instead of writing a return statement, we simply write the statement that we want to return. The “a + b” expression is an implicit return as the arrow function automatically returns it.
Use of Parentheses When Returning an Object
When using an implicit return with an arrow function to return an object, it’s essential to wrap the object with parentheses. This is because arrow functions interpret curly braces as a code block instead of an object literal.
Here’s an example of using an implicit return with an arrow function to return an object. const getPerson = (name, age) => ({
name: name,
In the example above, we’re returning an object with the “name” and “age” properties.
However, we wrap the object with parentheses to ensure that the arrow function returns an object literal instead of a code block.
Option to Turn off Eslint Rule with Comment
When using an implicit return with an arrow function, it’s also important to note that the Eslint rule might throw a warning or error. This is because some developers may find it confusing to read implicit returns, and they are not always intuitive in complex function bodies.
However, if a developer still prefers to use implicit returns despite the warning or error, they can turn off the Eslint rule with a comment. Here’s an example of turning off the Eslint rule with a comment.
/* eslint-disable arrow-body-style */
const multiplyNumbers = (a, b) => a * b;
In the example above, we disable the Eslint rule with the comment “/* eslint-disable arrow-body-style */”. This allows us to use implicit returns without Eslint throwing any warning or error messages.
In conclusion, using an implicit return with an arrow function is an alternative approach to solving the “Expected an assignment or function call and instead saw an expression” error when working with React.js. It simplifies the code and makes it more concise.
When returning an object with an implicit return, it’s important to wrap the object with parentheses. Finally, if the Eslint rule throws a warning or error when using implicit returns with arrow functions, developers can turn off the rule with a comment.
With these techniques, developers can choose the approach that works best for them and achieve efficient and reliable React.js applications. In summary, React.js developers often encounter the “Expected an assignment or function call and instead saw an expression” error, which can result from forgetting to return a value from a function or not explicitly returning an object when using the Array.map() method.
To resolve the issue, developers can use either an explicit return statement or implicit return with an arrow function. The latter approach provides a cleaner and more concise syntax for returning values, though it requires parentheses when returning an object.
Additionally, developers can use comments to disable Eslint rules that might flag implicit returns and limit readability. By being mindful of these solutions, React.js developers can avoid this common error and create efficient and reliable applications.
Remember to choose the solution that works best for your code and preferences.
Popular Posts
Resolving the java char cannot be dereferenced error: solutions and examples, transforming plain urls into clickable links with javascript, unlock the power of java classes: inheritance synchronization and more.
- Terms & Conditions
- Privacy Policy
no-unused-expressions
Disallow unused expressions
An unused expression which has no effect on the state of the program indicates a logic error.
For example, n + 1; is not a syntax error, but it might be a typing mistake where a programmer meant an assignment statement n += 1; instead. Sometimes, such unused expressions may be eliminated by some build tools in production environment, which possibly breaks application logic.
Rule Details
This rule aims to eliminate unused expressions which have no effect on the state of the program.
This rule does not apply to function calls or constructor calls with the new operator, because they could have side effects on the state of the program.
This rule does not apply to directives (which are in the form of literal string expressions such as "use strict"; at the beginning of a script, module, or function).
Sequence expressions (those using a comma, such as a = 1, b = 2 ) are always considered unused unless their return value is assigned or used in a condition evaluation, or a function call is made with the sequence expression value.
This rule, in its default state, does not require any arguments. If you would like to enable one or more of the following you may pass an object with the options set as follows:
- allowShortCircuit set to true will allow you to use short circuit evaluations in your expressions (Default: false ).
- allowTernary set to true will enable you to use ternary operators in your expressions similarly to short circuit evaluations (Default: false ).
- allowTaggedTemplates set to true will enable you to use tagged template literals in your expressions (Default: false ).
- enforceForJSX set to true will flag unused JSX element expressions (Default: false ).
These options allow unused expressions only if all of the code paths either directly change the state (for example, assignment statement) or could have side effects (for example, function call).
Examples of incorrect code for the default { "allowShortCircuit": false, "allowTernary": false } options:
Examples of correct code for the default { "allowShortCircuit": false, "allowTernary": false } options:
Note that one or more string expression statements (with or without semi-colons) will only be considered as unused if they are not in the beginning of a script, module, or function (alone and uninterrupted by other statements). Otherwise, they will be treated as part of a “directive prologue”, a section potentially usable by JavaScript engines. This includes “strict mode” directives.
Examples of correct code for this rule in regard to directives:
Examples of incorrect code for this rule in regard to directives:

allowShortCircuit
Examples of incorrect code for the { "allowShortCircuit": true } option:
Examples of correct code for the { "allowShortCircuit": true } option:
allowTernary
Examples of incorrect code for the { "allowTernary": true } option:
Examples of correct code for the { "allowTernary": true } option:
allowShortCircuit and allowTernary
Examples of correct code for the { "allowShortCircuit": true, "allowTernary": true } options:
allowTaggedTemplates
Examples of incorrect code for the { "allowTaggedTemplates": true } option:
Examples of correct code for the { "allowTaggedTemplates": true } option:
enforceForJSX
JSX is most-commonly used in the React ecosystem, where it is compiled to React.createElement expressions. Though free from side-effects, these calls are not automatically flagged by the no-unused-expression rule. If you’re using React, or any other side-effect-free JSX pragma, this option can be enabled to flag these expressions.
Examples of incorrect code for the { "enforceForJSX": true } option:
Examples of correct code for the { "enforceForJSX": true } option:
This rule was introduced in ESLint v0.1.0.
- Rule source
- Tests source
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[no-unused-expressions] Expected an assignment or function call and instead saw an expression: no-unused-expressions #531
Sampath-Lokuge commented Jun 7, 2021
rafaelss95 commented Jun 7, 2021
Sorry, something went wrong.
No branches or pull requests
- View all errors
- JSLint errors
- JSHint errors
- ESLint errors
- View all options
- JSLint options
- JSHint options
- ESLint options
Expected an assignment or function call and instead saw an expression
When do i get this error.
The "Expected an assignment or function call and instead saw an expression" error is thrown when JSLint, JSHint or ESLint encounters an expression with no effect . In the following example we have a conditional expression that will evaluate to true but has no other effect on the program:
Why do I get this error?
This error is raised to highlight a piece of useless and unnecessary code . The code will work as expected but since a lone floating expression has no effect on anything there is no point in it being there at all.
In general you would expect to see a statement which has an effect, such as assigning a value to a variable or invoking a function:
In JSHint 1.0.0 and above you have the ability to ignore any warning with a special option syntax . The identifier of this warning is W030 . This means you can tell JSHint to not issue this warning with the /*jshint -W030 */ directive.
In ESLint the rule that generates this warning is named no-unused-expressions . You can disable it by setting it to 0 , or enable it by setting it to 1 .
About the author
This article was written by James Allardice, Software engineer at Tesco and orangejellyfish in London. Passionate about React, Node and writing clean and maintainable JavaScript. Uses linters (currently ESLint) every day to help achieve this.
Follow me on Twitter or Google+
This project is supported by orangejellyfish , a London-based consultancy with a passion for JavaScript. All article content is available on GitHub under the Creative Commons Attribution-ShareAlike 3.0 Unported licence.
Have you found this site useful?
Please consider donating to help us continue writing and improving these articles.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Ternary Operator in Programming
- Unary Operators in Programming
- Types of Operators in Programming
- What are Operators in Programming?
- Binary Operators in Programming
- Ternary Operators in Solidity
- PHP | Ternary Operator
- Bitwise OR Operator (|) in Programming
- Logical OR operator in Programming
- Conditional Operator in Programming
- Logical AND operator in Programming
- Logical NOT Operator in Programming
- Bitwise AND operator in Programming
- Comparison Operators in Programming
- Assignment Operators in Programming
- C++ Ternary or Conditional Operator
- Operator Associativity in Programming
- Ternary Operator in Python
- Ternary Search for Competitive Programming
- Conditional or Ternary Operator (?:) in C
The Ternary Operator is similar to the if-else statement as it follows the same algorithm as of if-else statement but the ternary operator takes less space and helps to write the if-else statements in the shortest way possible. It is known as the ternary operator because it operates on three operands.
Table of Content
What is a Ternary Operator?
- Syntax of Ternary Operator
- Working of Ternary Operator
- Flowchart of Conditional/Ternary Operator
- Ternary Operator in C
- Ternary Operator in C++
- Ternary Operator in Java
- Ternary Operator in C#
- Ternary Operator in Javascript
- Applications of Ternary Operator
- Best Practices of Ternary Operator
The ternary operator is a conditional operator that takes three operands: a condition, a value to be returned if the condition is true , and a value to be returned if the condition is false . It evaluates the condition and returns one of the two specified values based on whether the condition is true or false.
Syntax of Ternary Operator:
The Ternary operator can be in the form
It can be visualized into an if-else statement as:
Working of Ternary Operator :
The working of the Ternary operator is as follows:
- Step 1: Expression1 is the condition to be evaluated.
- Step 2A: If the condition( Expression1 ) is True then Expression2 will be executed.
- Step 2B : If the condition( Expression1 ) is false then Expression3 will be executed.
- Step 3: Results will be returned
Flowchart of Conditional/Ternary Operator:
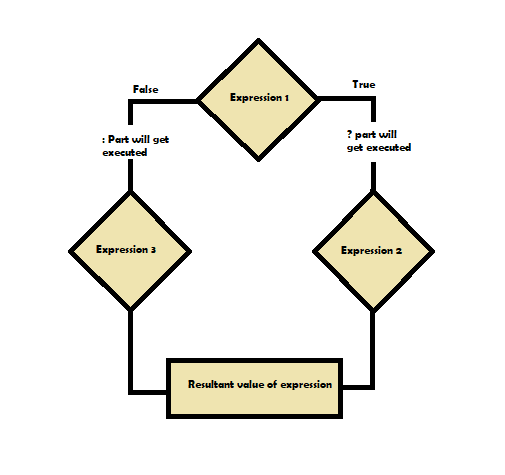
Flowchart of ternary operator
Ternary Operator in C:
Here are the implementation of Ternary Operator in C language:
Ternary Operator in C++:
Here are the implementation of Ternary Operator in C++ language:
Ternary Operator in Java:
Here are the implementation of Ternary Operator in java language:
Ternary Operator in Python:
Here are the implementation of Ternary Operator in python language:
Ternary Operator in C#:
Here are the implementation of Ternary Operator in C# language:
Ternary Operator in Javascript:
Here are the implementation of Ternary Operator in javascript language:
Applications of Ternary Operator:
- Assigning values based on conditions.
- Returning values from functions based on conditions.
- Printing different messages based on conditions.
- Handling null or default values conditionally.
- Setting properties or attributes conditionally in UI frameworks.
Best Practices of Ternary Operator:
- Use ternary operators for simple conditional expressions to improve code readability.
- Avoid nesting ternary operators excessively, as it can reduce code clarity.
- Use parentheses to clarify complex conditions and expressions involving ternary operators.
Conclusion:
The conditional operator or ternary operator is generally used when we need a short conditional code such as assigning value to a variable based on the condition. It can be used in bigger conditions but it will make the program very complex and unreadable.
Please Login to comment...
Similar reads.
- Programming
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

COMMENTS
I believe this is because the ternary operator evaluates the expression and returns a value that is expected to be assigned. For example: var test = (my_var > 0) ? true : false; However, you are using it like a regular if/then/else statement. While the ternary operator does perform if/then/else, it is traditionally used in assignents.
Secondly, you should use "Conditional (ternary) Operator". Share. Improve this answer. Follow edited Aug 4, 2021 at 12:35. Luis Paulo Pinto. 5,818 4 4 gold badges 22 22 silver badges 36 36 bronze badges. answered ... Expected an assignment or function call and instead saw an expression - React. 0.
The code for this article is available on GitHub. We solved the issue in our map() method by explicitly returning. This is necessary because the Array.map () method returns an array containing all of the values that were returned from the callback function we passed to it. Note that when you return from a nested function, you aren't also ...
Inside the function, we use the ternary operator to check if the number is even or odd. If the number modulo 2 equals 0 (meaning it's divisible by 2 with no remainder), then the condition evaluates to true, and the string "even" is assigned to the result variable. If the condition evaluates to false (meaning the number is odd), the string "odd ...
Expected an assignment or function call and instead saw an expression. Could any one point out where I've gone wrong? ... 2019, 12:13pm 2. The ternary is just sitting there. You forgot to return it. You could even drop the ternary and just return the condition. 1 Like. najccforum June 19, 2019, 12:18pm 3. Doh! thanks very much .
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as an alternative to an if ...
Step 1: Open the terminal and type the following command to create a react app. change the <folder-name> to whatever you want, and press enter. Step 2: Open the folder inside the code editor, and delete all the files inside the "src" folder except the index.js file. Step 3: Delete all the files inside the public folder.
React.js Error: "Expected an assignment or function call and instead saw an expression" React.js is an open-source, front-end JavaScript library utilized for building user interfaces and UI components. With its ability to create reusable UI components, React.js has become a favorite tool for developers. However, like any language-based tool, React.js has its own sets of […]
Why does my ternary operator return an error: Expected an assignment or function call and instead saw an expression, but not my if statement? I'll add more code if need be, but essentially, I'm trying to return className if a condition is true, and do nothing if the condition is false.
The main problem is that one leg of the ternary is a function call, and the other is an expression. I'd say it's the reason ESLint complains and I'm pretty sure no warning would appear if instead of '' he'd put a different function call. Last, agreed on the best option being an if here, or a && operator.
This rule does not apply to directives (which are in the form of literal string expressions such as "use strict"; at the beginning of a script, module, or function).. Sequence expressions (those using a comma, such as a = 1, b = 2) are always considered unused unless their return value is assigned or used in a condition evaluation, or a function call is made with the sequence expression value.
Hi, Why can't I use Ternary with 2 statements here? res?.isSuccessful ? (this.toastService.showToast(res.message, 'success'), this.queueDataService.addMember(attendee ...
In such case it is always possible to use a function call, but this can be cumbersome and inelegant. For example, to pass conditionally different values as an argument for a constructor of a field or a base class, it is impossible to use a plain if-else statement; in this case we can use a conditional assignment expression, or a function call ...
In general you would expect to see a statement which has an effect, such as assigning a value to a variable or invoking a function: var x = 1; x = 2; // Assignment instead of unused expression. In JSHint 1.0.0 and above you have the ability to ignore any warning with a special option syntax. The identifier of this warning is W030 .
Currently getting Expected an assignment or function call and instead saw an expression no-unused-expressions I tried returning an empty div at the end of my ternary operator but still get the s...
replace food in your ternary operator with foodType and remove foodType from result1 and result2 and replace them with strings "Mexican" : "other";
Explanation. The function call operator provides function semantics for any object.. The conditional operator (colloquially referred to as ternary conditional) checks the boolean value of the first expression and, depending on the resulting value, evaluates and returns either the second or the third expression. [] Built-in function call operatoThe function call expressions have the form
Expected an assignment or function call and instead saw an expression. Now I don't want to just disable the rule, but I don't see anything inherently wrong with the code. ... There are various ways to refactor this to look more like a statement, like replacing the ternary operator with an if statement, or by moving the function up to the top level.
While the ternary operator is a way of re-writing a classic if-else block, in a certain sense, it behaves like a function since it returns a value. Indeed, we can assign the result of this operation to a variable: my_var = a if condition else b. For example: x = "Is true" if True else "Is false" print(x) Is true.
conditional ternary operator is giving error: Expected an assignment or function call and instead saw an expression when setting a variable Hot Network Questions "No obligation cash offer on your house" What is the risk
What is a Ternary Operator? The ternary operator is a conditional operator that takes three operands: a condition, a value to be returned if the condition is true, and a value to be returned if the condition is false.It evaluates the condition and returns one of the two specified values based on whether the condition is true or false.